Converting CSV to JSON in JavaScript: A Comprehensive Guide
In the world of data manipulation, converting CSV (Comma-Separated Values) files to JSON (JavaScript Object Notation) is a frequent requirement. JSON is a versatile data format that’s easy to work with in JavaScript. This guide will equip you with the knowledge and skills needed to perform this conversion efficiently.
Prerequisites
Before diving into the conversion process, ensure you have the following prerequisites in place:
- Basic knowledge of JavaScript;
- Node.js installed on your system;
- Text editor or integrated development environment (IDE).
Method 1: Using CSVtoJSON Library
CSVtoJSON is a popular JavaScript library that simplifies CSV to JSON conversion. It’s an excellent choice for developers looking for a hassle-free solution.
To get started, install the library using npm:

Once installed, you can use the library to convert CSV to JSON with just a few lines of code:
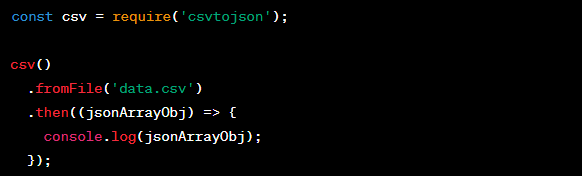
This method provides a quick and efficient way to convert CSV data to JSON.
Method 2: Manual Conversion
If you prefer more control over the conversion process, you can manually convert CSV to JSON in JavaScript. Here’s a step-by-step approach:
Step 1: Read the CSV File

Step 2: Parse CSV Data

Step 3: Convert to JSON
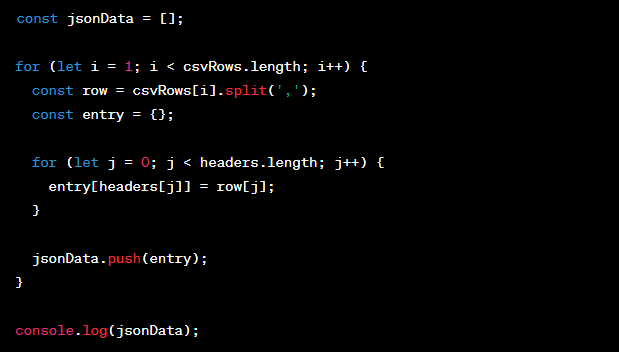
This method allows for custom data manipulation during the conversion process.
Handling Data Errors
When converting data, it’s essential to anticipate and handle errors gracefully. You can add error handling to your conversion code to ensure smooth processing.
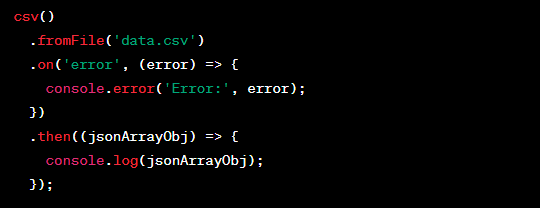
Adding Data Validation
Data validation is crucial to ensure the integrity of your JSON data. You can validate fields during the conversion process to catch and correct errors.
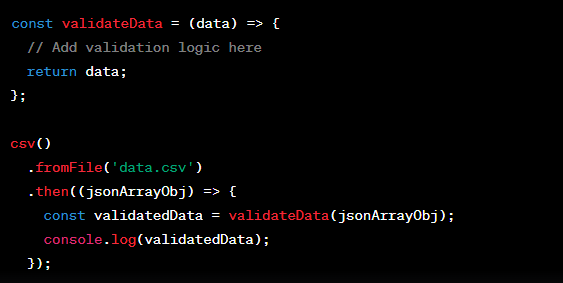
Optimizing Performance
Optimizing performance is vital when dealing with large CSV files. Consider using streaming techniques and asynchronous processing to handle substantial datasets efficiently.
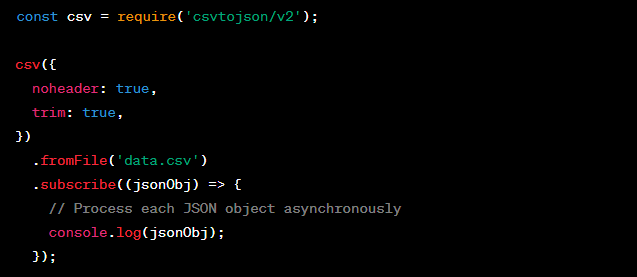
Real-World Use Cases
Let’s explore some real-world scenarios where CSV to JSON conversion plays a pivotal role:
- Importing data from spreadsheets into web applications;
- Transforming data for data analysis and visualization;
- Migrating data between different systems and databases.
Best Practices
To ensure a seamless CSV to JSON conversion experience, follow these best practices:
- Keep your code modular and well-structured;
- Handle errors and edge cases gracefully;
- Test your conversion process with various CSV file formats;
- Consider data validation and sanitization;
- Optimize for performance, especially with large datasets.
Conclusion
Converting CSV to JSON in JavaScript is a valuable skill for any developer working with data. Whether you choose to use a library like CSVtoJSON or implement a custom solution, this guide has provided you with the knowledge and tools to perform the conversion efficiently.
FAQs
CSV is a plain-text format for tabular data, while JSON is a data interchange format that can represent structured data with nested objects and arrays.
Yes, you can convert JSON data back to CSV format by writing custom code to iterate through the JSON objects and generate CSV rows.
Processing large CSV files may require optimizing memory usage and using streaming techniques to avoid performance issues.
Yes, you can automate the conversion process in a web application by allowing users to upload CSV files and using JavaScript to perform the conversion on the server-side.
Yes, libraries like jsonschema and ajv can be used for JSON schema validation in JavaScript.
JavaScript Remove Accents: Simplify Text Handling
In the world of data manipulation, converting CSV (Comma-Separated Values) files to JSON (JavaScript Object Notation) is a frequent requirement. JSON is a versatile data format that’s easy to work with in JavaScript. This guide will equip you with the knowledge and skills needed to perform this conversion efficiently. Prerequisites Before diving into the conversion …
The difference between for…in, for…of and forEach?
In the world of data manipulation, converting CSV (Comma-Separated Values) files to JSON (JavaScript Object Notation) is a frequent requirement. JSON is a versatile data format that’s easy to work with in JavaScript. This guide will equip you with the knowledge and skills needed to perform this conversion efficiently. Prerequisites Before diving into the conversion …