How to Define an Enum in JavaScript: A Comprehensive Guide
JavaScript, known for its flexibility, offers various ways to define enumerations (enums) to represent a fixed set of values. Enums are particularly useful when you want to maintain a collection of related constants in your code. In this guide, we will delve into the world of enums in JavaScript, covering essential concepts, practical examples, and best practices.
Let’s start our journey by understanding the basics.
Understanding Enums
What Are Enums?
Enums, short for enumerations, are a data type that allows you to define a set of named constant values. These constants are usually related and represent specific states or options in your application. By using enums, you can enhance code readability and maintainability.
The Need for Enums
Why use enums in JavaScript? Consider a scenario where you need to represent the days of the week. Without enums, you might use plain strings or numbers, making your code less intuitive and prone to errors. Enums offer a more structured and descriptive approach.
Enum Declaration
In JavaScript, enums can be declared in several ways. The most common method is by using Object literals or the Object.freeze method. Let’s explore both approaches.
Using Object Literals
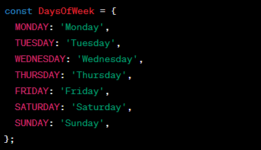
Using Object.freeze
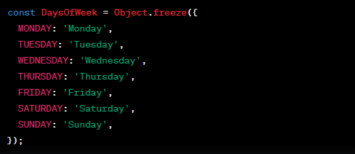
Enum Values
Once you’ve defined an enum, you can access its values using dot notation:

Enums are essentially objects with named properties, and their values are immutable.
Enum Use Cases
Enums find applications in various scenarios. Let’s explore a few use cases to understand their significance.
1. Role-Based Access Control
Imagine you’re building an application with role-based access control. You can use enums to define user roles:
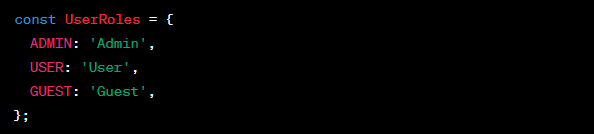
This makes it clear and efficient to manage user permissions and roles.
2. Days of the Week
As mentioned earlier, representing days of the week is a classic use case for enums. It simplifies your code and improves readability when dealing with scheduling or calendar-related tasks.
3. HTTP Status Codes
HTTP status codes, such as 404 (Not Found) or 200 (OK), are another example. Enums help maintain a consistent and understandable way to handle network responses.
Enum Best Practices
To ensure your enum implementation is effective, consider these best practices:
- Use Descriptive Names: Choose meaningful names for your enum constants to enhance code clarity. Avoid ambiguous or generic names;
- Prevent Modifications: Freeze your enum objects using Object.freeze to prevent accidental modifications. This ensures that the enum values remain constant throughout your application’s lifecycle;
- Document Your Enums: Always provide comments or documentation to explain the purpose and usage of your enums. This helps other developers understand your code quickly.
Enum vs. Alternative Approaches
Enum vs. Plain Objects
While you can use plain objects to achieve similar results, enums offer a more semantic and standardized approach. They explicitly declare the intended purpose of the values.
Enum vs. Arrays
Arrays can also store a collection of values, but they lack the specificity and immutability that enums provide. Enum values cannot be accidentally modified or reordered.
Implementing Enums in Real Projects
To illustrate the practical use of enums, let’s consider an example where we define an enum for HTTP methods.
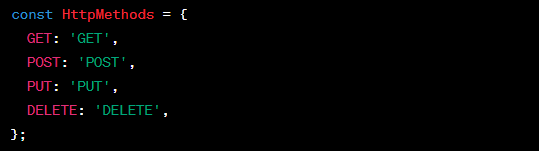
Now, in your API request handling code, you can use this enum to ensure valid HTTP method usage:
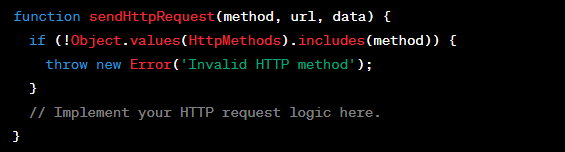
Conclusion
In this extensive guide, we’ve explored the concept of enums in JavaScript, from their definition to practical use cases and best practices. Enums offer a powerful tool for enhancing code readability and maintainability in your projects. By following the guidelines provided here, you can effectively implement enums and improve the overall quality of your JavaScript code.
FAQs
Enums are a standard part of modern JavaScript and are supported in all major browsers and Node.js environments. However, if you need to support older browsers, consider transpiling your code with tools like Babel.
While we commonly associate enums with string values, you can also use them for numerical values. Just ensure that the values you assign are unique within the enum.
Enums have minimal performance overhead and are generally as efficient as plain objects. They are a suitable choice for most use cases.
To explore advanced enum techniques and broader JavaScript topics, consider referring to official documentation, online tutorials, and JavaScript community forums.
Implementing a Timeout in JavaScript Promises
JavaScript, known for its flexibility, offers various ways to define enumerations (enums) to represent a fixed set of values. Enums are particularly useful when you want to maintain a collection of related constants in your code. In this guide, we will delve into the world of enums in JavaScript, covering essential concepts, practical examples, and …
JavaScript Remove Accents: Simplify Text Handling
JavaScript, known for its flexibility, offers various ways to define enumerations (enums) to represent a fixed set of values. Enums are particularly useful when you want to maintain a collection of related constants in your code. In this guide, we will delve into the world of enums in JavaScript, covering essential concepts, practical examples, and …