The difference between for…in, for…of and forEach?
JavaScript, the versatile language powering the web, offers various ways to iterate through data. Among these, the for…in, for…of, and forEach loops are essential tools for handling collections and arrays. In this comprehensive guide, we will explore the differences between these loops, when to use them, and why they matter in modern JavaScript development.
The Basics of Iteration
Before delving into the specifics, let’s briefly understand the concept of iteration in JavaScript.
What is Iteration?
Iteration is the process of repeating a set of actions or instructions for each element in a collection or array. It allows developers to perform tasks like accessing array elements, manipulating data, and executing code repeatedly.
Common Use Cases for Iteration
- Looping through Arrays: Iteration helps process every element in an array, performing actions on each item as needed;
- Iterating over Object Properties: Objects in JavaScript can also be iterated to access their key-value pairs.
Now, let’s embark on a journey to explore the differences between for…in, for…of, and forEach loops.
The for…in Loop
Overview
The for…in loop is primarily used for iterating over the properties of an object. It loops through the enumerable properties of an object, including inherited properties.
Syntax

Example
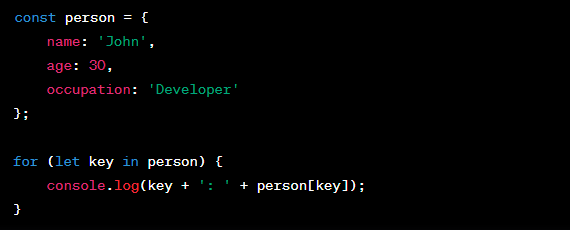
Key Points
- Iterates over object properties;
- Not suitable for iterating through arrays or iterable objects;
- May include inherited properties if not used with proper checks.
The for…of Loop
Overview
The for…of loop is designed for iterating through iterable objects like arrays, strings, maps, and sets. It provides a simple and concise way to access elements.
Syntax

Example

Key Points
- Tailored for iterating through iterable objects;
- Ensures a clean and straightforward syntax;
- Does not work with object properties.
The forEach Loop
Overview
The forEach loop is specifically designed for arrays. It provides an elegant way to iterate through array elements and execute a provided function for each element.
Syntax
Example
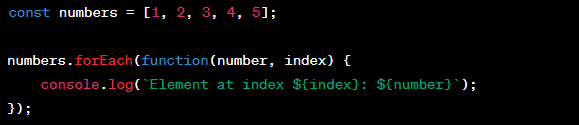
Key Points
- Ideal for iterating through arrays;
- Simplifies the syntax for iterating and applying functions;
- Cannot be used with objects or other iterable types.
Let’s summarize the key differences between these three loops in a comparison table:
Aspect | for…in | for…of | forEach |
---|---|---|---|
Suitable for arrays | No | Yes | Yes |
Suitable for objects | Yes | No | No |
Inherited properties | May include | N/A | N/A |
Syntax complexity | Simple | Very simple | Simple |
Use cases | Object properties | Iterable objects | Arrays |
Which One to Choose?
The choice between for…in, for…of, and forEach depends on your specific use case:
- Use for…in when you need to iterate through an object’s properties, including inherited ones;
- Use for…of when you want to loop through iterable objects like arrays, strings, maps, or sets;
- Use forEach when working exclusively with arrays and you need a clean and concise syntax for iteration.
Common Pitfalls
While these loops are powerful tools, developers often make mistakes when choosing the wrong loop for the job. Here are some common pitfalls:
- Using for…in with Arrays: Avoid using for…in loops with arrays, as they can include unexpected properties;
- Using forEach for Non-Arrays: Remember that forEach is only for arrays; using it on other objects will result in errors;
- Skipping Object Checks: When using for…in with objects, always check if the property is the object’s own property to avoid unexpected results.
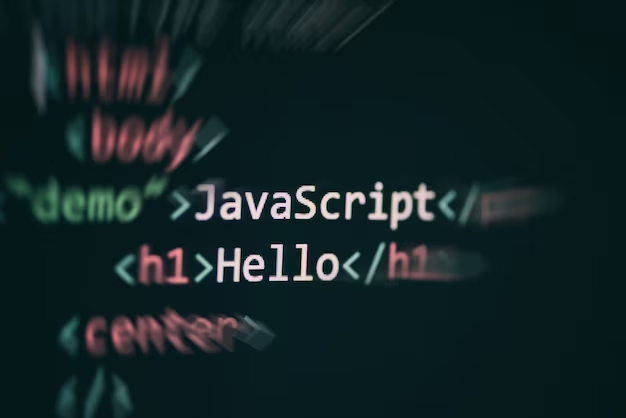
Conclusion
In this in-depth exploration, we’ve dissected the differences between JavaScript’s for…in, for…of, and forEach loops. Understanding when and how to use these loops is essential for efficient coding and data manipulation in JavaScript. Choose the loop that best suits your needs, and watch your code become more concise and readable.
FAQs
Yes, there can be performance differences depending on the use case. forEach is often the slowest, while for…of tends to be faster for arrays.
No, for…of is designed for iterable objects like arrays and strings. It won’t work with regular objects.
It’s a good practice to use hasOwnProperty() when using for…in with objects to avoid iterating over inherited properties.
Yes, for…in, for…of, and forEach are supported in modern JavaScript environments, including browsers and Node.js.
Yes, you can nest these loops to perform more complex iterations, but be cautious as it can lead to code that’s harder to maintain.
Converting CSV to JSON in JavaScript: A Comprehensive Guide
JavaScript, the versatile language powering the web, offers various ways to iterate through data. Among these, the for…in, for…of, and forEach loops are essential tools for handling collections and arrays. In this comprehensive guide, we will explore the differences between these loops, when to use them, and why they matter in modern JavaScript development. The …
Comparing Two Objects in JavaScript: A Step-by-Step Guide
JavaScript, the versatile language powering the web, offers various ways to iterate through data. Among these, the for…in, for…of, and forEach loops are essential tools for handling collections and arrays. In this comprehensive guide, we will explore the differences between these loops, when to use them, and why they matter in modern JavaScript development. The …