JavaScript: Is It Pass-by-Value or Pass-by-Reference?
JavaScript employs a pass-by-value mechanism for managing data types, treating everything as a value type by default. However, this straightforward approach takes an intriguing twist when it encounters objects.
The intrigue arises from the fact that objects are considered reference types, yet they are passed by value. While this may initially appear contradictory, it’s crucial to understand that when you pass an object to a function, what you’re actually passing is a reference to that object, and this reference is passed by value. The key distinction to grasp here is that passing an object reference by value is distinct from passing the object itself by reference.
To simplify this concept, consider that any changes made to the object within a function will have a direct impact on the original object because both the object and its reference within the function point to the same object in memory. However, modifying the value of the variable initially holding the object will not affect the object referenced within the function. To clarify this intricate behavior, let’s explore it through a practical example:
let myObj = { a: 1 };
const myFunc = obj => {
obj.a++;
return obj;
}
let otherObj = myFunc(myObj);
myObj; // { a: 2 }
otherObj; // { a: 2 }
myObj === otherObj; // true
myObj = { a: 4, b: 0 };
myObj; // { a: 4, b: 0 }
otherObj; // { a: 2 }
myObj === otherObj; // false
In this illustrative scenario, we delve into the world of JavaScript with a plain object named myObj, which takes center stage as an argument within the myFunc function. Within the confines of myFunc, the obj parameter plays a pivotal role as it points directly to the same object, myObj. Consequently, any adjustments made to obj reverberate directly onto myObj, as both variables are tethered to the very same object residing in memory. This results in the intriguing outcome where assigning the function’s result (represented by obj) to another variable, say otherObj, essentially bequeaths this new variable the exact same reference. As a result, both myObj and otherObj become synchronized and manipulate the identical object’s value.
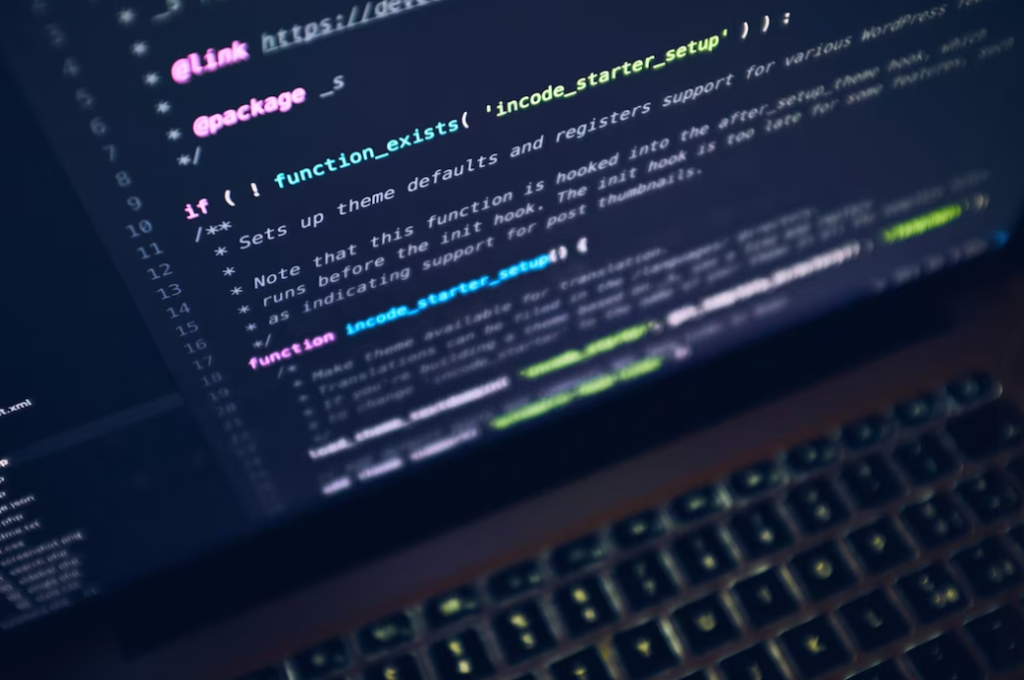
However, it’s imperative to emphasize that when myObj undergoes a reassignment to a new object value, this transformation doesn’t ripple through to affect otherObj in any way. otherObj steadfastly maintains its reference to the original object. This observation underscores a fundamental facet of JavaScript’s behavior: it operates on a pass-by-value mechanism, decisively distinct from a pass-by-reference paradigm. This illumination makes it clear that altering myObj has no bearing on `otherObj,’ offering a clear delineation of JavaScript’s intrinsic pass-by-value nature.
Conclusion
JavaScript’s variable handling can be perplexing, but this comprehensive guide has clarified the matter. JavaScript employs a pass-by-value approach for all data types, treating them as value types. However, objects introduce a unique aspect. Although objects are reference types, they are still passed by value, meaning a reference to the object is passed to a function, not the object itself. This leads to situations where changes within a function directly affect the original object. When assigning the function’s result to another variable, both variables share the same reference, synchronizing with the object’s value. Nevertheless, reassigning the original variable doesn’t affect the function’s reference. This underlines that JavaScript operates on a pass-by-value basis, not pass-by-reference, which is essential knowledge for effective JavaScript development.
JavaScript: Understanding Sync & Async Code
JavaScript employs a pass-by-value mechanism for managing data types, treating everything as a value type by default. However, this straightforward approach takes an intriguing twist when it encounters objects. The intrigue arises from the fact that objects are considered reference types, yet they are passed by value. While this may initially appear contradictory, it’s crucial …
A Detailed Guide on JS Sleep Function Alternatives
JavaScript employs a pass-by-value mechanism for managing data types, treating everything as a value type by default. However, this straightforward approach takes an intriguing twist when it encounters objects. The intrigue arises from the fact that objects are considered reference types, yet they are passed by value. While this may initially appear contradictory, it’s crucial …