A Guide to Appending Elements in JavaScript Arrays
Appending a value or values from an array in JavaScript is a frequently encountered task. Although it is not particularly challenging, there are several methods at your disposal, each with its own advantages and disadvantages. The choice of the most suitable method ultimately hinges on the specific use case.
Array.prototype.push()
The conventional approach for adding elements to the end of an array involves employing Array.prototype.push(). Although it offers versatility, it’s important to keep in mind that this method modifies the original array. Conversely, it provides the capability to add multiple elements simultaneously. Notably, the return value of Array.prototype.push() is the updated length of the array.
const arr = ['a', 'b', 'c'];
arr.push('d', 'e'); // Returns 5 (new length after appending 2 elements)
// arr = ['a', 'b', 'c', 'd', 'e']
Array.prototype.unshift()
Much like Array.prototype.push(), Array.prototype.unshift() adds elements to the beginning of an array. Additionally, this approach alters the original array and enables the simultaneous addition of multiple elements.
const arr = ['a', 'b', 'c'];
arr.unshift('d', 'e'); // Returns 5 (new length after appending 2 elements)
// arr = ['d', 'e', 'a', 'b', 'c']
Array.prototype.splice()
Array.prototype.splice() is commonly employed for removing elements from an array, but it can also serve to append elements when needed. Despite its capability to modify the original array, it offers flexibility by allowing the addition of elements at any position within the array. Like the earlier methods, it supports the simultaneous addition of multiple elements. In this particular use-case, the return value of this method is essentially inconsequential.
const arr = ['a', 'b', 'c'];
arr.splice(1, 0, 'd', 'e');
// arr = ['a', 'd', 'e', 'b', 'c']
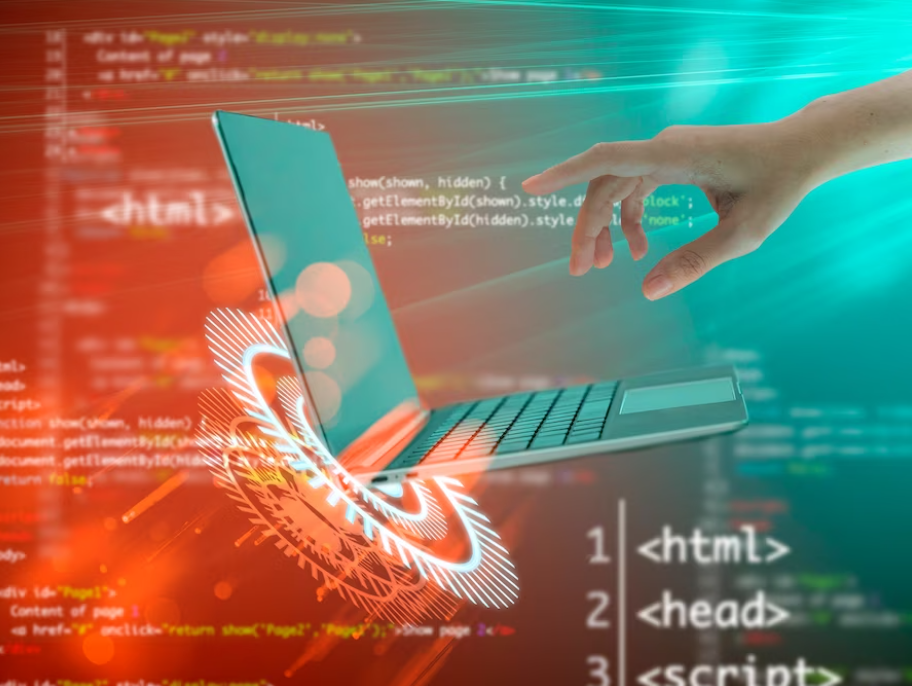
Array.prototype.length
When you want to append an element to the end of an array, you set a value for an index that is equal to the array’s length. This is a consequence of JavaScript arrays being zero-indexed. Fortunately, you can achieve this by combining Array.prototype.length with array index notation. Like the methods mentioned earlier, this technique modifies the original array. It’s worth noting that this method allows the addition of only one element at a time, and it can only be added to the end of the array.
const arr = ['a', 'b', 'c'];
arr[arr.length] = 'd';
// arr = ['a', 'b', 'c', 'd']
Array.prototype.concat()
The initial choice that maintains the original array’s integrity is Array.prototype.concat(). This method generates a fresh array by concatenating the elements of the original array with the new elements. It offers the flexibility to append elements to either the beginning or the end of the array, and it also permits the addition of multiple elements simultaneously.
const arr = ['a', 'b', 'c'];
const arr2 = arr.concat('d', 'e');
// arr = ['a', 'b', 'c'], arr2 = ['a', 'b', 'c', 'd', 'e']
const arr3 = ['d', 'e'].concat(...arr);
// arr = ['a', 'b', 'c'], arr3 = ['d', 'e', 'a', 'b', 'c']
Spread operator
Lastly, the ultimate choice for preserving the original array’s state involves using the spread operator (…). It functions in a manner similar to Array.prototype.concat(), facilitating the appending of one or multiple elements to either the beginning or the end of the array.
const arr = ['a', 'b', 'c'];
const arr2 = [...arr, 'd', 'e'];
// arr = ['a', 'b', 'c'], arr2 = ['a', 'b', 'c', 'd', 'e']
const arr3 = ['d', 'e', ...arr];
// arr = ['a', 'b', 'c'], arr3 = ['d', 'e', 'a', 'b', 'c']
Right Method for Appending Elements to a JavaScript Array
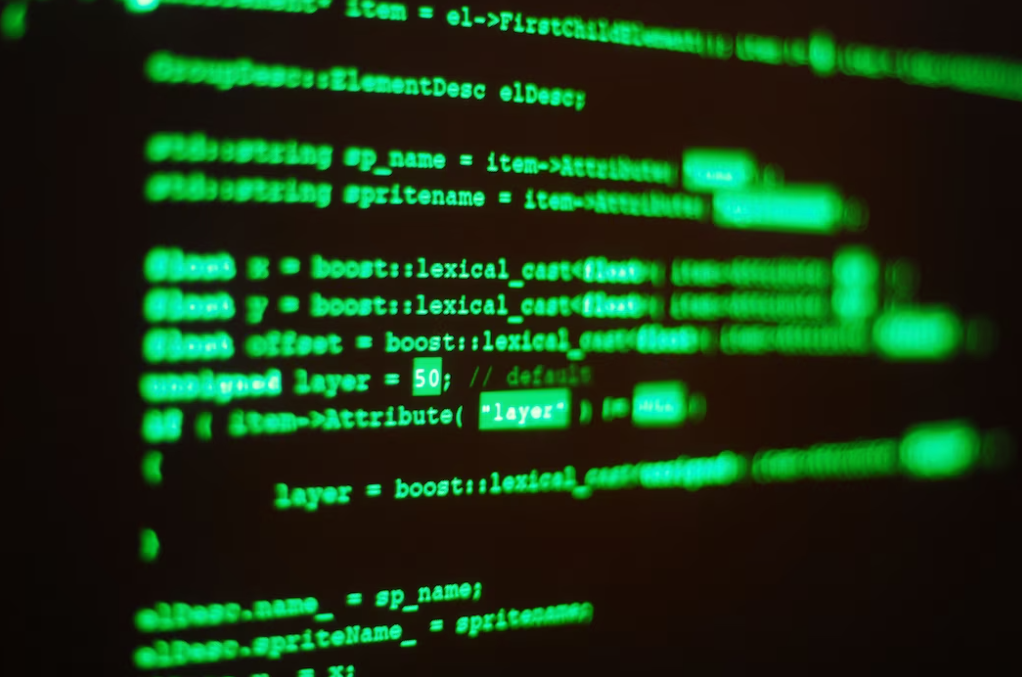
When it comes to appending elements to a JavaScript array, it’s essential to select the right method for the job. In this comprehensive guide on JavaScript array manipulation, we’ve explored various techniques, each with its own set of advantages and considerations. Whether you need to add elements to the beginning or end of an array, or you require more control over their placement, understanding the nuances of Array.prototype.push(), Array.prototype.unshift(), Array.prototype.splice(), Array.prototype.length, Array.prototype.concat(), and the spread operator (…) is crucial. By making informed choices based on your specific coding needs, you can enhance your JavaScript skills and write more efficient and maintainable code.
Conclusion
Appending elements to a JavaScript array involves various methods, each suited to specific needs. Array.prototype.push() and Array.prototype.unshift() are ideal for simple additions at the array’s start or end. If you require more control, Array.prototype.splice() allows flexible placement. Array.prototype.length is straightforward for appending at the end, and Array.prototype.concat() offers versatile array creation. Lastly, the spread operator (…) is a modern, efficient way to maintain the original array while appending elements. Familiarity with these methods empowers developers to make informed choices and enhance their JavaScript array manipulation skills.
Comparing HTMLElement.innerText vs. Node.textContent
Appending a value or values from an array in JavaScript is a frequently encountered task. Although it is not particularly challenging, there are several methods at your disposal, each with its own advantages and disadvantages. The choice of the most suitable method ultimately hinges on the specific use case. Array.prototype.push() The conventional approach for adding …
Exploring JavaScript’s Asynchronous Array Iteration
Appending a value or values from an array in JavaScript is a frequently encountered task. Although it is not particularly challenging, there are several methods at your disposal, each with its own advantages and disadvantages. The choice of the most suitable method ultimately hinges on the specific use case. Array.prototype.push() The conventional approach for adding …