JavaScript Array Data: Replacing or Appending Values
Learn how to efficiently replace or append values in a JavaScript array using the spread operator and Array.prototype methods. This comprehensive guide covers the process of creating a shallow copy of an array, finding the index of elements, and performing replacements or appends with ease. Boost your coding skills and optimize your arrays today!
Array Element Update Techniques in JavaScript
Updates an element in an array or adds it if it’s not present:
- Begin by creating a shallow copy of the array using the spread operator (…);
- Employ the Array.prototype.findIndex() method to locate the index of the first element meeting the criteria set by the comparison function, compFn;
- If no matching element is discovered, utilize Array.prototype.push() to add the new value to the end of the array;
- Alternatively, if a matching element is found, utilize Array.prototype.splice() to substitute the value at the located index with the new value.
const replaceOrAppend = (arr, val, compFn) => {
const res = [...arr];
const i = arr.findIndex(v => compFn(v, val));
if (i === -1) res.push(val);
else res.splice(i, 1, val);
return res;
};
Example:
const people = [ { name: 'John', age: 30 }, { name: 'Jane', age: 28 } ];
const jane = { name: 'Jane', age: 29 };
const jack = { name: 'Jack', age: 28 };
replaceOrAppend(people, jane, (a, b) => a.name === b.name);
// [ { name: 'John', age: 30 }, { name: 'Jane', age: 29 } ]
replaceOrAppend(people, jack, (a, b) => a.name === b.name);
// [
// { name: 'John', age: 30 },
// { name: 'Jane', age: 28 },
// { name: 'Jack', age: 28 }
// ]
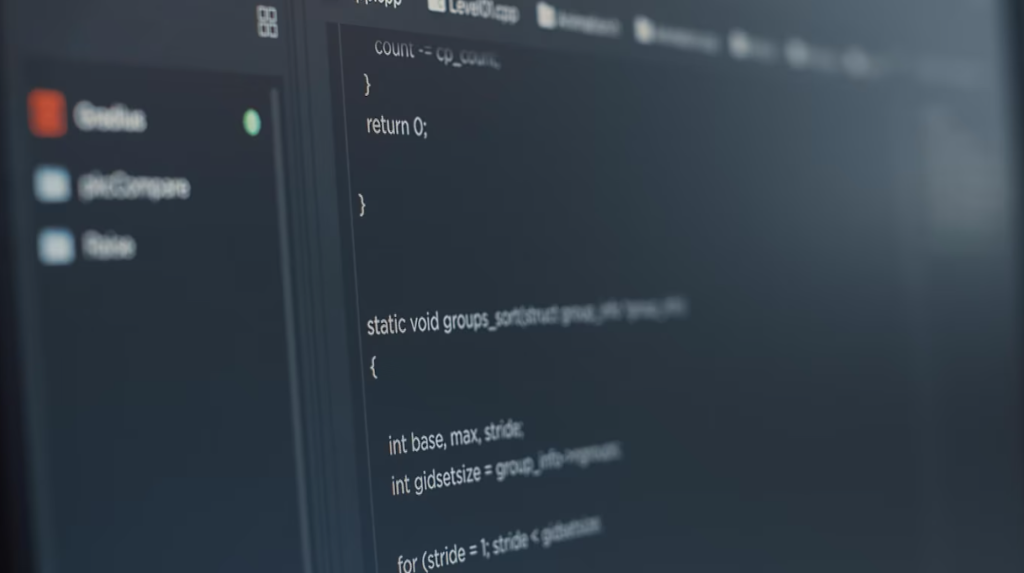
Conclusion
Mastering array manipulation in JavaScript is essential for optimizing code. By creating shallow copies, using findIndex(), push(), and splice(), you can efficiently update or append elements, enhancing code flexibility. This knowledge optimizes JavaScript projects, making them more maintainable and adaptable, and equips you for array manipulation challenges, boosting your coding skills.
Why NaN Stands Alone in Javascript’s Inequality Test
Learn how to efficiently replace or append values in a JavaScript array using the spread operator and Array.prototype methods. This comprehensive guide covers the process of creating a shallow copy of an array, finding the index of elements, and performing replacements or appends with ease. Boost your coding skills and optimize your arrays today! Array …
JavaScript: Object to Map Transformation
Learn how to efficiently replace or append values in a JavaScript array using the spread operator and Array.prototype methods. This comprehensive guide covers the process of creating a shallow copy of an array, finding the index of elements, and performing replacements or appends with ease. Boost your coding skills and optimize your arrays today! Array …