JavaScript: Object to Map Transformation
JavaScript is a versatile and dynamic programming language widely used for web development. One common task in JavaScript is converting objects into different data structures for various purposes. One such conversion is transforming objects into maps. In this article, we will explore why you might want to convert objects to maps, how to do it, and some practical use cases.
Why Convert Objects to Maps?
Property | Maps | Objects |
---|---|---|
Preservation of Property Order | Guaranteed to maintain key order | No guaranteed order |
Key Flexibility | Support keys of any data type | Limited to string and symbol keys |
Iteration Simplicity | Built-in methods for iteration (e.g., forEach, entries) | Manual iteration using for…in loops or Object methods (e.g., Object.keys()) |
No Prototype Pollution | Maps do not have prototype pollution issues | Potential for prototype pollution issues |
JS objects and maps have some similarities, but they also have distinct differences that make one more suitable than the other for specific use cases.
- Preservation of Property Order: Maps guarantee that the order of keys is maintained, whereas objects traditionally do not. This property makes maps preferable when you need to iterate over keys in a specific order;
- Key Flexibility: Objects in JavaScript only allow string and symbol keys, while maps can have keys of any data type, including objects and functions. This flexibility can be advantageous when working with complex data structures;
- Iteration Simplicity: Maps provide built-in methods like forEach, entries, keys, and values that make it easier to iterate over their content. Objects require manual iteration using for…in loops or object methods like Object.keys();
- No Prototype Pollution: Converting objects to maps can help avoid prototype pollution, a security issue where unintended properties or methods are added to an object’s prototype chain. Maps don’t have this concern.
Converting Objects to Maps
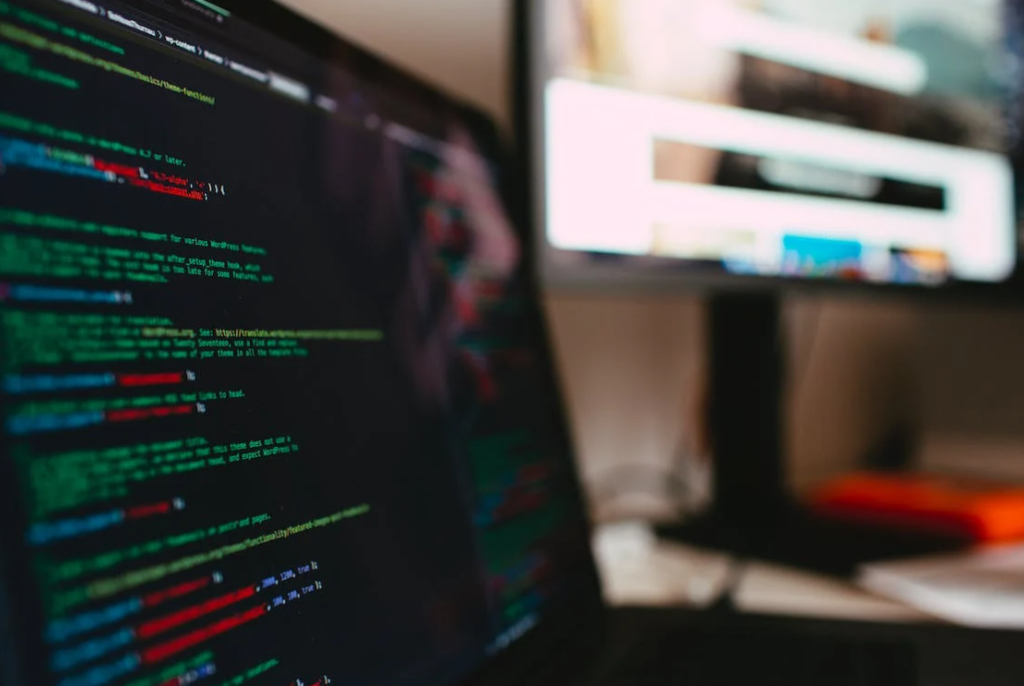
Transforms an object into a Map.
- Utilize Object.entries() for the conversion of the object into an array of key-value pairs;
- Employ the Map constructor to transform the array into a Map.
const objectToMap = obj => new Map(Object.entries(obj));
Examples:
objectToMap({a: 1, b: 2}); // Map {‘a’ => 1, ‘b’ => 2}
Conclusion
Mastering the conversion of JavaScript objects into maps is a valuable skill for any web developer. This step-by-step guide has elucidated the reasons why this transformation might be necessary, highlighting the benefits of preserving property order, key flexibility, iteration simplicity, and the avoidance of prototype pollution. The provided code snippet, which employs the Object.entries() method and the Map constructor, offers a straightforward and efficient way to accomplish this conversion. By understanding when and how to convert objects to maps, developers can enhance their data manipulation capabilities and optimize their code for a wide range of practical use cases in web development.
JavaScript Array Data: Replacing or Appending Values
JavaScript is a versatile and dynamic programming language widely used for web development. One common task in JavaScript is converting objects into different data structures for various purposes. One such conversion is transforming objects into maps. In this article, we will explore why you might want to convert objects to maps, how to do it, …
JavaScript’s encodeURI() and encodeURIComponent()
JavaScript is a versatile and dynamic programming language widely used for web development. One common task in JavaScript is converting objects into different data structures for various purposes. One such conversion is transforming objects into maps. In this article, we will explore why you might want to convert objects to maps, how to do it, …