JavaScript Naming Conventions: Step-by-Step Guide
When it comes to writing clean and maintainable JavaScript code, adhering to naming conventions is essential. Proper naming not only makes your code more readable but also helps you communicate your intentions effectively to other developers. In this guide, we’ll dive into JavaScript naming conventions, covering variables, functions, constants, classes, and the use of prefixes for private elements.
Variables
- Case Sensitivity: In JavaScript, variable names are case-sensitive. This means that myVariable and myvariable are considered different variables;
- Start with a Letter: Variable names should always start with a letter (a-z or A-Z);
- CamelCase: Use camelCase for naming variables. This convention capitalizes the first letter of each word except the first one. For example, myVariableName is in camelCase;
- Descriptive Names: Make your variable names self-descriptive, indicating the purpose or content of the variable. For instance, instead of x, use userAge to represent the age of a user;
- Boolean Variables: When naming boolean variables, it’s common to prefix them with “is” or “has.” For example, isActive, hasPermission, or isLoaded.
Functions
- Case Sensitivity: Function names in JavaScript are also case-sensitive;
- Start with a Letter: Function names should begin with a letter;
- CamelCase: Like variables, use camelCase for function names;
- Descriptive Names: Function names should be descriptive and typically use verbs in the imperative form. For example, calculateTotal, getUserData, or validateInput;
- Common Prefixes: Common prefixes for function names include “get” (for accessing data), “make” (for creating something), “apply” (for performing an action), and so on;
- Class Methods: Methods within JavaScript classes follow the same naming conventions as regular functions.
Constants
- Case Sensitivity: Constants are case-sensitive in JavaScript;
- Define at the Top: It’s a good practice to define constants at the top of your file, function, or class to make them easily accessible and maintainable;
- Naming Convention: Constants can be named using either UPPER_SNAKE_CASE (all uppercase with underscores) or plain camelCase, depending on your project’s conventions. Choose one style and stick with it for consistency.
Classes
- Case Sensitivity: Class names are also case-sensitive;
- Start with a Capital Letter: Class names should start with a capital letter, and the convention for naming classes is PascalCase, where each word’s first letter is capitalized. For example, Person, ProductManager, or ShoppingCart;
- Descriptive Names: Class names should provide a clear understanding of the class’s purpose and functionality. Choose meaningful and descriptive names;
- Components in Frontend Frameworks: When working with frontend frameworks like React or Angular, components follow the same naming rules as regular JavaScript classes.
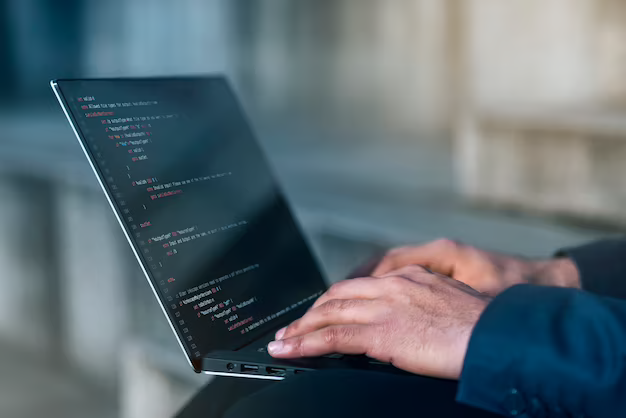
JavaScript Naming Conventions: A Quick Comparison
To make it easier to understand the JavaScript naming conventions, let’s compare different naming styles for variables, functions, and classes.
Element | Convention | Example |
---|---|---|
Variables | camelCase | myVariableName |
Functions | camelCase | getUserData |
Constants | UPPER_SNAKE_CASE | MAX_LENGTH |
Classes | PascalCase | Person, UserModel |
Private | _underscore_prefix | _privateVariable |
These conventions ensure code consistency and readability while communicating the purpose of each element effectively.
Conclusion
In the world of JavaScript, adhering to naming conventions is crucial for writing clean and maintainable code. Consistency in a variable, function, class, and constant names not only makes your code more readable but also helps in collaborative development. Following these naming conventions will lead to a smoother coding experience, whether you’re working on personal projects or contributing to larger codebases.
Remember, while these conventions are widely accepted best practices, it’s essential to follow the specific guidelines of the project you’re working on. Consistency within a codebase is often more critical than strict adherence to any one convention.
FAQ
While it’s generally a good practice to follow established naming conventions, you can use different conventions if they align better with your project or team’s preferences. The key is to maintain consistency within your codebase.
CamelCase starts with a lowercase letter and capitalizes the first letter of each subsequent word (e.g., myVariableName). PascalCase, on the other hand, starts with an uppercase letter and capitalizes the first letter of each subsequent word (e.g., MyClassName). CamelCase is typically used for variable and function names, while PascalCase is used for class and constructor names.
Self-descriptive variable names make your code more understandable. When someone else reads your code, or when you revisit it after some time, descriptive names provide context about the purpose and usage of the variable.
Common prefixes for function names include “get” (for retrieving data), “make” (for creating new instances), “apply” (for applying actions), and others. These prefixes help indicate the function’s purpose.
No, JavaScript doesn’t have built-in support for private variables or functions. Using the “_” prefix is a convention to signal that a variable or function should be considered private, but it doesn’t prevent access to them. Developers should exercise caution and not access these variables or functions directly to maintain encapsulation.
Understanding Binary Trees in JavaScript
When it comes to writing clean and maintainable JavaScript code, adhering to naming conventions is essential. Proper naming not only makes your code more readable but also helps you communicate your intentions effectively to other developers. In this guide, we’ll dive into JavaScript naming conventions, covering variables, functions, constants, classes, and the use of prefixes …
Comprehensive Guide: Initializing JavaScript Arrays
When it comes to writing clean and maintainable JavaScript code, adhering to naming conventions is essential. Proper naming not only makes your code more readable but also helps you communicate your intentions effectively to other developers. In this guide, we’ll dive into JavaScript naming conventions, covering variables, functions, constants, classes, and the use of prefixes …