Switching to Object Literals for Cleaner JavaScript Code
JavaScript, one of the most popular programming languages in the world, provides various tools and constructs to make your code more efficient and readable. However, one area that often perplexes developers is the use of switch statements for handling conditional logic. While switch statements can be useful, they can also lead to bloated, hard-to-maintain code. In this article, we’ll explore a cleaner and more modern alternative: using object literals to replace JavaScript switch statements.
The Problem with Switch Statements
Switch statements are a traditional way to handle multiple conditions in JavaScript. They work by evaluating an expression and then executing code based on matching case labels. While they serve their purpose, switch statements come with some drawbacks:
- Verbosity: Switch statements can quickly become verbose and cluttered when handling numerous cases. Each case requires a separate block of code, making the structure less intuitive;
- Lack of Flexibility: Switch statements are rigid and don’t allow for dynamic case labels or complex conditional expressions, limiting their versatility;
- Potential for Errors: Typographical errors, such as missing ‘break’ statements, can lead to unexpected behavior, making debugging a challenge.
Transitioning from Switch to Object Literals
JavaScript’s switch statement can be a bit challenging to recall due to its syntax. Fortunately, tools like VS Code’s autocomplete come to the rescue. However, it does stand out syntactically by not using curly braces, and the need for ‘break’ statements in every case can be error-prone. Additionally, its procedural control flow can impact performance.
Fortunately, JavaScript offers a compelling alternative in the form of object literals for most switch statement scenarios. The concept is simple: create an object with keys representing the cases you’d have in a switch statement. Then, you can directly access the desired value using the expression you would typically pass to the switch statement. This approach streamlines your code and improves its readability.
let fruit = 'oranges';
switch (fruit) {
case 'apples':
console.log('Apples');
break;
case 'oranges':
console.log('Oranges');
break;
}
// Logs: 'Oranges'
const logFruit = {
'apples': () => console.log('Apples'),
'oranges': () => console.log('Oranges')
};
logFruit[fruit](); // Logs: 'Oranges'
Although this approach greatly enhances readability and reduces verbosity, its speed improvement is substantial as well. Nevertheless, we must tackle a crucial aspect: the default case. To address it, we can simply introduce a ‘default’ key and verify if the expression’s value exists within our object.
let fruit = 'strawberries';
switch (fruit) {
case 'apples':
console.log('Apples');
break;
case 'oranges':
console.log('Oranges');
break;
default:
console.log('Unknown fruit');
}
// Logs: 'Unknown fruit'
const logFruit = {
'apples': () => console.log('Apples'),
'oranges': () => console.log('Oranges'),
'default': () => console.log('Unknown fruit')
};
(logFruit[fruit] || logFruit['default'])(); // Logs: 'Unknown fruit'
Finally, the object literal replacement should effectively handle falling-through cases, mirroring the behavior observed when no ‘break’ statement is present. Achieving this simply involves extracting and reusing logic within the object literal.
let fruit = 'oranges';
switch (fruit) {
case 'apples':
case 'oranges':
console.log('Known fruit');
break;
default:
console.log('Unknown fruit');
}
// Logs: 'Known fruit'
const knownFruit = () => console.log('Known fruit');
const unknownFruit = () => console.log('Unknown fruit');
const logFruit = {
'apples': knownFruit,
'oranges': knownFruit,
'default': unknownFruit
};
(logFruit[fruit] || logFruit['default'])(); // Logs: 'Known fruit'
To conclude, we can consolidate and abstract this logic into a reusable function. This function will accept the lookup object and an optional name for the default case (we’ll use _default by default to prevent conflicts). Subsequently, this function will return another function with the applicable lookup logic, allowing us to replace switch statements effortlessly.
const switchFn = (lookupObject, defaultCase = '_default') =>
expression => (lookupObject[expression] || lookupObject[defaultCase])();
const knownFruit = () => console.log('Known fruit');
const unknownFruit = () => console.log('Unknown fruit');
const logFruit = {
'apples': knownFruit,
'oranges': knownFruit,
'default': unknownFruit
};
const fruitSwitch = switchFn(logFruit, 'default');
fruitSwitch('apples'); // Logs: 'Known fruit'
fruitSwitch('pineapples'); // Logs: 'Unknown fruit'
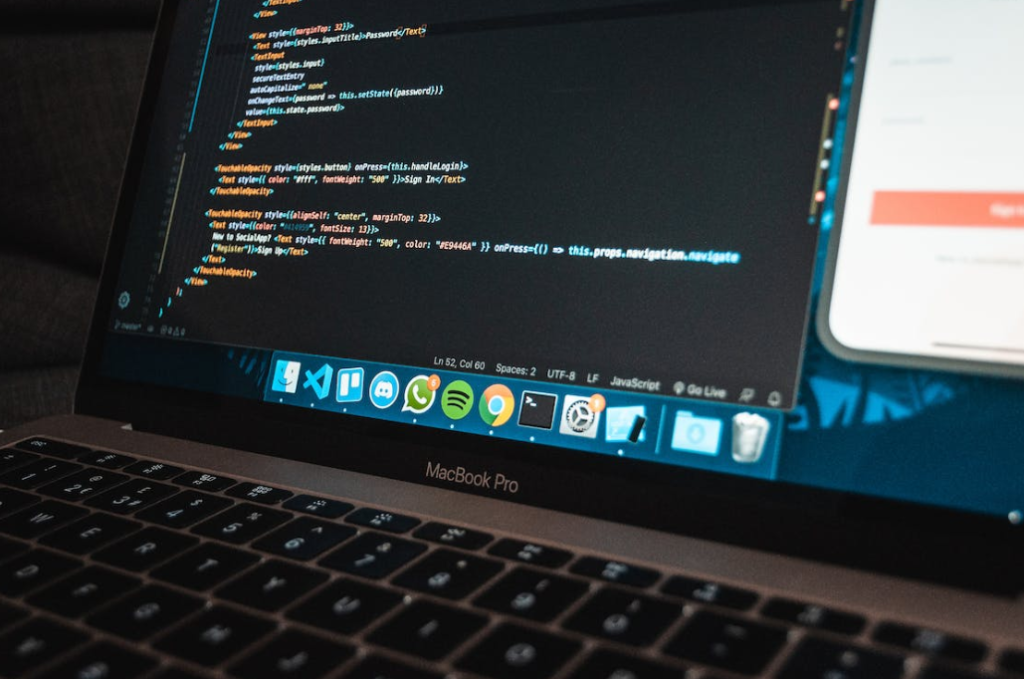
Benefits of Using Object Literals
Replacing switch statements with object literals offers several advantages:
Aspect | Description |
---|---|
Readability | Object literals provide a clear and concise representation of conditional logic, enhancing code readability and comprehension. |
Maintainability | Centralizing all conditions and actions within a single object simplifies making changes or additions, reducing the likelihood of errors and facilitating code maintenance. |
Extensibility | Object literals offer ease of extension for accommodating new conditions or actions, ensuring a scalable and organized codebase. |
Error Prevention | By minimizing the risk of common switch statement errors such as missing ‘break’ statements or duplicate cases, object literals contribute to error prevention and code robustness. |
Conclusion
Transitioning from JavaScript switch statements to object literals enhances code clarity and maintainability. While switch statements can be verbose and rigid, object literals offer an elegant and flexible alternative. Object literals simplify code, reduce the risk of errors, and handle default cases and falling-through scenarios effectively. To simplify adoption, encapsulating the logic into a reusable function is practical. Embracing object literals and this function can significantly improve your JavaScript development, fostering more maintainable code and leaving behind switch statement complexities.
JavaScript’s encodeURI() and encodeURIComponent()
JavaScript, one of the most popular programming languages in the world, provides various tools and constructs to make your code more efficient and readable. However, one area that often perplexes developers is the use of switch statements for handling conditional logic. While switch statements can be useful, they can also lead to bloated, hard-to-maintain code. …
Enhance JSON Object Formatting with JavaScript
JavaScript, one of the most popular programming languages in the world, provides various tools and constructs to make your code more efficient and readable. However, one area that often perplexes developers is the use of switch statements for handling conditional logic. While switch statements can be useful, they can also lead to bloated, hard-to-maintain code. …