JavaScript: Understanding Sync & Async Code
JavaScript, a versatile and widely used programming language, offers developers the flexibility to choose between synchronous and asynchronous coding approaches when designing web applications. These two paradigms play a pivotal role in determining how tasks are executed and how user experiences are managed. In this article, let’s delve into the fundamental differences between synchronous and asynchronous code in JavaScript and explore when to use each approach.
Synchronous Code Execution
Sequential execution, characteristic of synchronous code, follows a step-by-step order. In this paradigm, each task waits for its predecessor to finish before commencing, ensuring a well-ordered progression of operations.
console.log('One');
console.log('Two');
console.log('Three');
// LOGS: 'One', 'Two', 'Three'
Pros:
- Simplicity: Synchronous code typically boasts easier readability and debugging due to its straightforward, linear execution flow;
- Predictability: Developers enjoy precise control over the execution order, guaranteeing that essential tasks are carried out sequentially.
Cons:
- Blocking: Synchronous operations have the potential to obstruct the entire program, resulting in unresponsiveness in web applications, particularly when handling time-consuming activities such as network requests or data processing;
- User Experience: Prolonged synchronous operations can diminish the user experience by introducing delays and unresponsiveness in web applications.
Asynchronous Code Execution
Asynchronous code execution empowers tasks to run independently and simultaneously. This means that operations can proceed without waiting for each other, fostering a non-blocking approach. JavaScript employs various mechanisms, such as callbacks, promises, and async/await, to achieve this concurrency and enhance program responsiveness.
Asynchronous code operates concurrently, allowing one operation to occur while another is still underway:
console.log('One');
setTimeout(() => console.log('Two'), 100);
console.log('Three');
// LOGS: 'One', 'Three', 'Two'
Asynchronous code execution finds its preference in scenarios where there is a possibility of operations causing indefinite blocking. This versatility applies to numerous contexts, encompassing network requests, computationally intensive tasks, interactions with the file system, and beyond. The integration of asynchronous code into web browsers plays a pivotal role in ensuring that web pages maintain their responsiveness. Consequently, this approach upholds an exceptional user experience, even when resource-intensive processes are underway, guaranteeing uninterrupted interaction and seamless navigation.
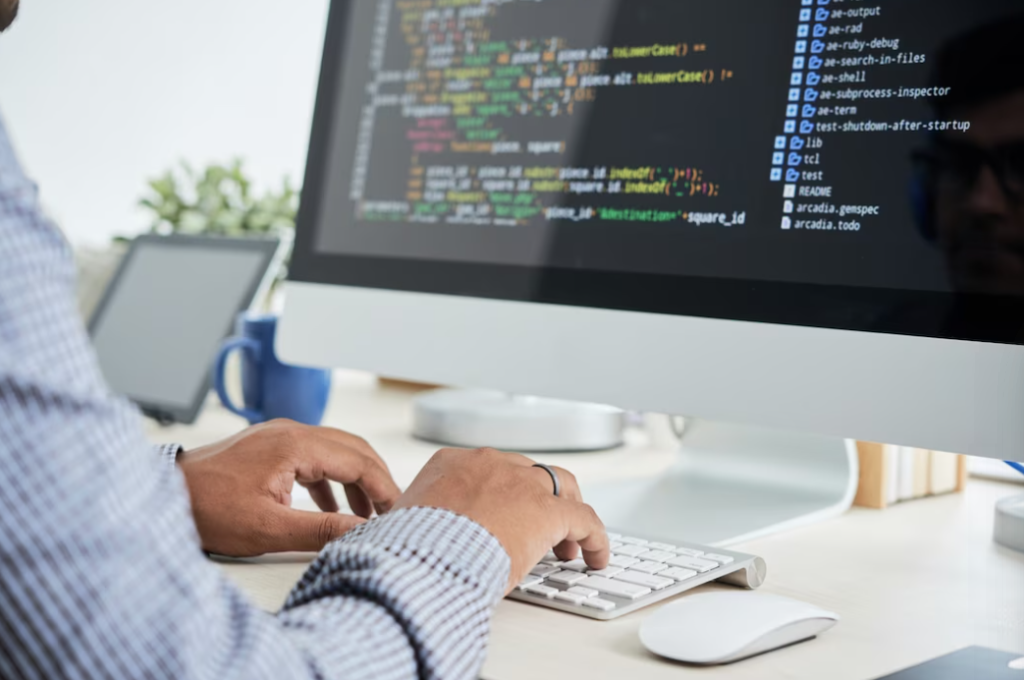
Pros:
- Non-blocking: Asynchronous operations don’t impede the program’s flow, making them well-suited for tasks like network requests and I/O operations;
- Enhanced Responsiveness: In web development, asynchronous code ensures that the user interface remains responsive even during resource-intensive operations;
- Efficiency: Asynchronous code can enhance resource utilization by enabling the program to execute multiple tasks concurrently.
Cons:
- Complexity: Managing asynchronous code can be more challenging, as it necessitates handling callbacks, promises, and potential race conditions;
- Debugging: Debugging asynchronous code can prove trickier due to its non-linear flow, making it harder to trace the sequence of events.
When to Use Synchronous vs. Asynchronous Code
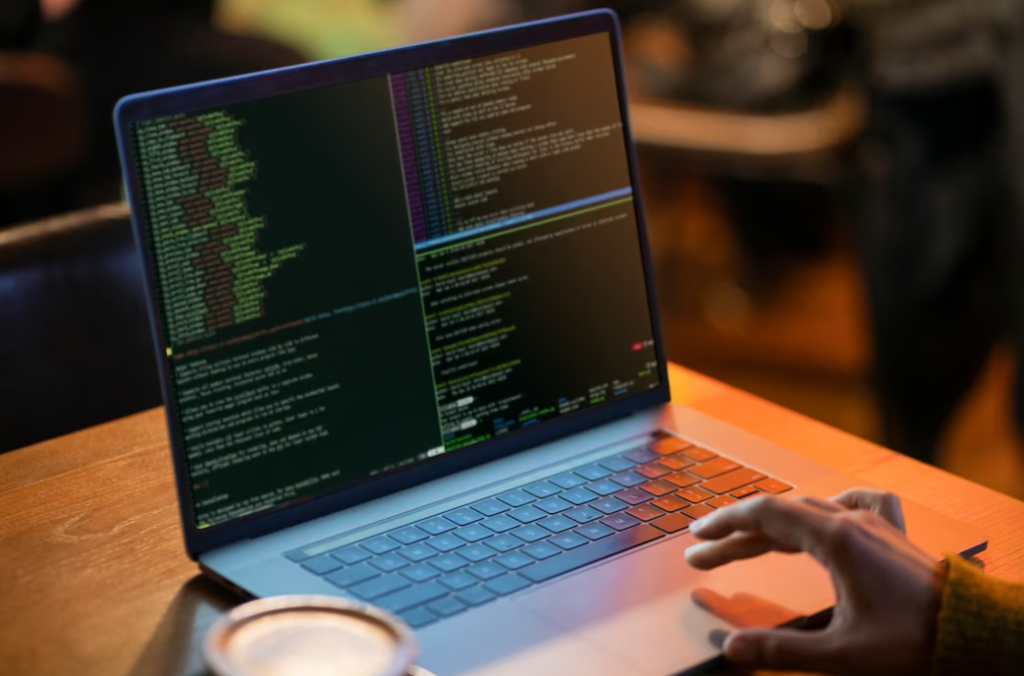
The choice between these codes depends on the specific use case:
Synchronous Code | Asynchronous Code |
---|---|
Suitable for tasks that require sequential execution. | Ideal for tasks involving I/O operations like network requests, file operations, and database interactions. |
Blocking the program’s flow is not a concern. | Ensures a non-blocking approach, allowing concurrent execution of tasks. |
Typically used for simple data processing. | Ensures a responsive user interface in web applications, even during resource-intensive operations. |
Operations are executed one after the other. | Operations can run independently, improving efficiency and preventing program lockups. |
Examples include basic data manipulation. | Examples include AJAX requests, reading/writing files, and handling multiple user interactions simultaneously. |
Conclusion
Understanding the distinction between synchronous and asynchronous code in JavaScript is pivotal for building efficient and responsive web applications. Synchronous code delivers simplicity and predictability but can lead to blocking issues. In contrast, asynchronous code provides non-blocking behavior and improved responsiveness, though it requires careful handling of concurrency. The choice between these approaches should be guided by your application’s specific requirements, aiming to achieve a well-rounded equilibrium between predictability and performance.
How to Check If a String Contains a Substring in JavaScript
JavaScript, a versatile and widely used programming language, offers developers the flexibility to choose between synchronous and asynchronous coding approaches when designing web applications. These two paradigms play a pivotal role in determining how tasks are executed and how user experiences are managed. In this article, let’s delve into the fundamental differences between synchronous …
JavaScript: Is It Pass-by-Value or Pass-by-Reference?
JavaScript, a versatile and widely used programming language, offers developers the flexibility to choose between synchronous and asynchronous coding approaches when designing web applications. These two paradigms play a pivotal role in determining how tasks are executed and how user experiences are managed. In this article, let’s delve into the fundamental differences between synchronous …