Exploring JavaScript’s Asynchronous Array Iteration
Navigating asynchronous operations can present a significant challenge for numerous developers, especially when these intricacies become interwoven with the process of looping through arrays. It’s important to note that each available approach carries its unique complexities and potential stumbling blocks, adding an extra layer of complexity to asynchronous programming.
For loops
Leveraging the synergy of asynchronous operations and for (or for…of) loops stands out as one of the simplest methods for handling asynchronous tasks with array elements in JavaScript. When you incorporate the “await” keyword within a for loop, a distinctive behavior takes place: the code pauses, patiently waiting for each asynchronous operation to finish before proceeding. This ensures that all promises execute sequentially, enabling a more controlled and predictable flow in your code.
const asyncUppercase = item =>
new Promise(resolve =>
setTimeout(
() => resolve(item.toUpperCase()),
Math.floor(Math.random() * 1000)
)
);
const uppercaseItems = async () => {
const items = ['a', 'b', 'c'];
for (item of items) {
const uppercaseItem = await asyncUppercase(item);
console.log(uppercaseItem);
}
console.log('Items processed');
};
uppercaseItems();
// LOGS: 'A', 'B', 'C', 'Items processed'
Promises
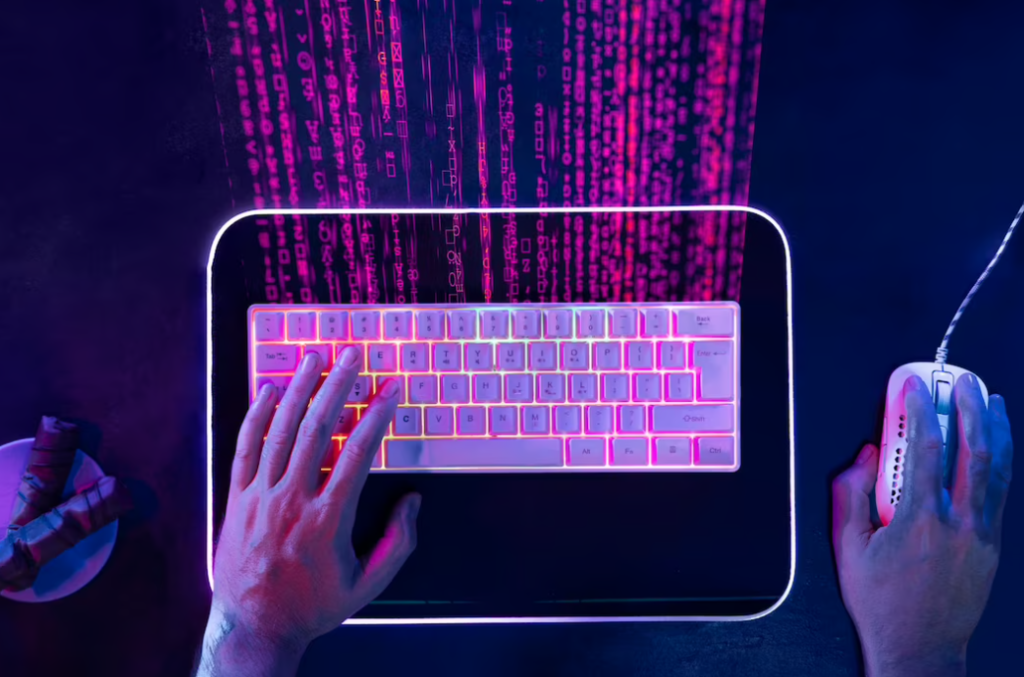
Promise.all() introduces an alternative approach to handling asynchronous loops over arrays. Its primary distinction from the previously mentioned method is that Promise.all() runs all asynchronous operations concurrently. This concurrent execution implies that promises may not complete in the same order they were initiated, a behavior that can introduce complications in specific scenarios.
More often than not, this parallel execution aligns with the desired behavior, as the need for promises to execute in a strict sequential order is relatively uncommon. This makes Promise.all() a preferred solution for many developers when working with asynchronous operations over arrays.
const asyncUppercase = item =>
new Promise(resolve =>
setTimeout(
() => resolve(item.toUpperCase()),
Math.floor(Math.random() * 1000)
)
);
const uppercaseItems = () => {
const items = ['a', 'b', 'c'];
return Promise.all(
items.map(async item => {
const uppercaseItem = await asyncUppercase(item);
console.log(uppercaseItem);
})
).then(() => {
console.log('Items processed');
});
};
// LOGS: 'A', 'C', 'B', 'Items processed'
Array methods
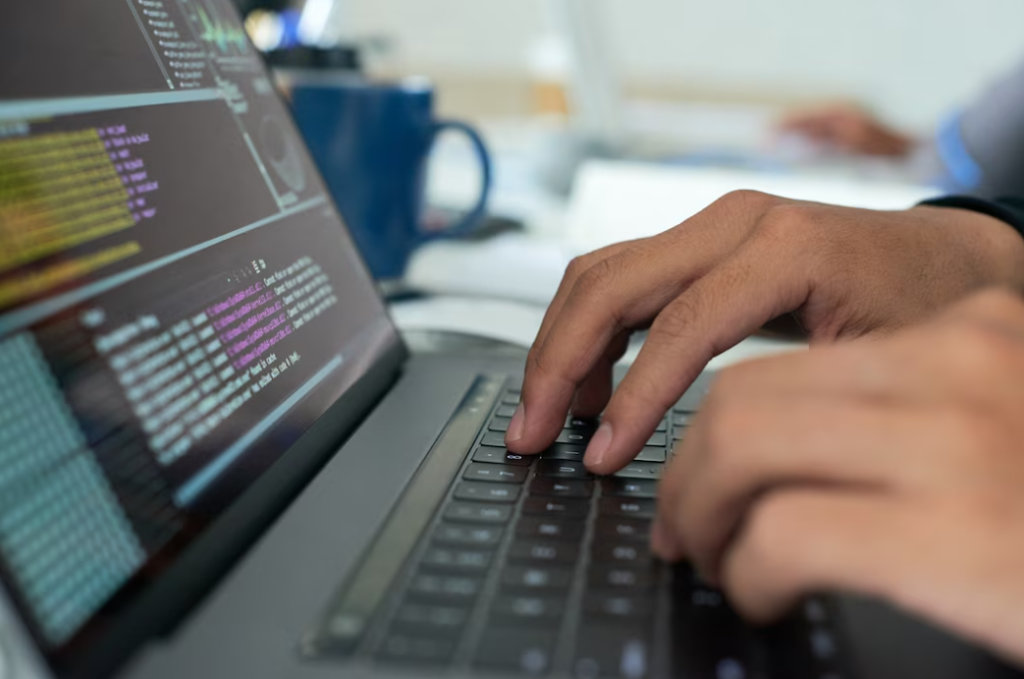
Regrettably, traditional array methods like Array.prototype.forEach() do not seamlessly integrate with async/await constructs. In such cases, the optimal and reliable approach is to resort to Promise.all(), as demonstrated in the earlier example. Attempting to use an async callback within Array.prototype.forEach() can lead to unintended consequences, with the rest of the code proceeding without awaiting the asynchronous operations.
const asyncUppercase = item =>
new Promise(resolve =>
setTimeout(
() => resolve(item.toUpperCase()),
Math.floor(Math.random() * 1000)
)
);
const uppercaseItems = async () => {
const items = ['a', 'b', 'c'];
await items.forEach(async item => {
const uppercaseItem = await asyncUppercase(item);
console.log(uppercaseItem);
});
console.log('Items processed');
};
uppercaseItems();
// LOGS: ''Items processed', 'B', 'A', 'C'
Conclusion
This comprehensive guide has shed light on the intricate world of asynchronous array loops in JavaScript, offering developers valuable insights into optimizing their code for increased efficiency and enhanced handling of complex data structures. We explored various methods, including the “for” loop, “Promise.all(),” and traditional array methods like “Array.prototype.forEach(),” each with its own unique advantages and caveats. While the “for” loop provides sequential execution for precise control, “Promise.all()” empowers concurrent processing, often aligning with the desired behavior. However, we caution against the use of async callbacks within traditional array methods, as they can lead to unintended consequences. Armed with this knowledge, developers can make informed decisions and unlock the potential of asynchronous array looping, ultimately taking their JavaScript coding skills to new heights.
A Guide to Appending Elements in JavaScript Arrays
Navigating asynchronous operations can present a significant challenge for numerous developers, especially when these intricacies become interwoven with the process of looping through arrays. It’s important to note that each available approach carries its unique complexities and potential stumbling blocks, adding an extra layer of complexity to asynchronous programming. For loops Leveraging the synergy of …
Implementing a Timeout in JavaScript Promises
Navigating asynchronous operations can present a significant challenge for numerous developers, especially when these intricacies become interwoven with the process of looping through arrays. It’s important to note that each available approach carries its unique complexities and potential stumbling blocks, adding an extra layer of complexity to asynchronous programming. For loops Leveraging the synergy of …