JavaScript’s encodeURI() and encodeURIComponent()
JavaScript is a versatile programming language that provides developers with a wide range of tools for working with strings and manipulating data. When dealing with web development and handling URLs, you often need to encode and decode special characters to ensure data integrity and security. Two commonly used functions for this purpose are encodeURI() and encodeURIComponent(). While they may seem similar at first glance, they serve different purposes and have distinct use cases. In this article, we’ll explore the differences between encodeURI() and encodeURIComponent() in JavaScript.
encodeURIComponent()
The encodeURIComponent() function encodes all characters within the provided string except for A-Z, a-z, 0-9, hyphen (-), underscore (_), period (.), exclamation mark (!), tilde (~), asterisk (*), single quotation mark (‘), and parentheses (). It is the recommended choice when you are encoding a portion of a URL rather than the entire URL itself.
const partOfURL = 'my-page#with,speci@l&/"characters"?';
const fullURL = 'https://my-website.com/my-page?query="a%b"&user=1';
encodeURIComponent(partOfURL); // Good, escapes special characters
// 'my-page%23with%2Cspeci%40l%26%2F%22characters%22%3F'
encodeURIComponent(fullURL); // Bad, encoded URL is not valid
// 'https%3A%2F%2Fmy-website.com%2Fmy-page%3Fquery%3D%22a%25b%22%26user%3D1'
encodeURI()
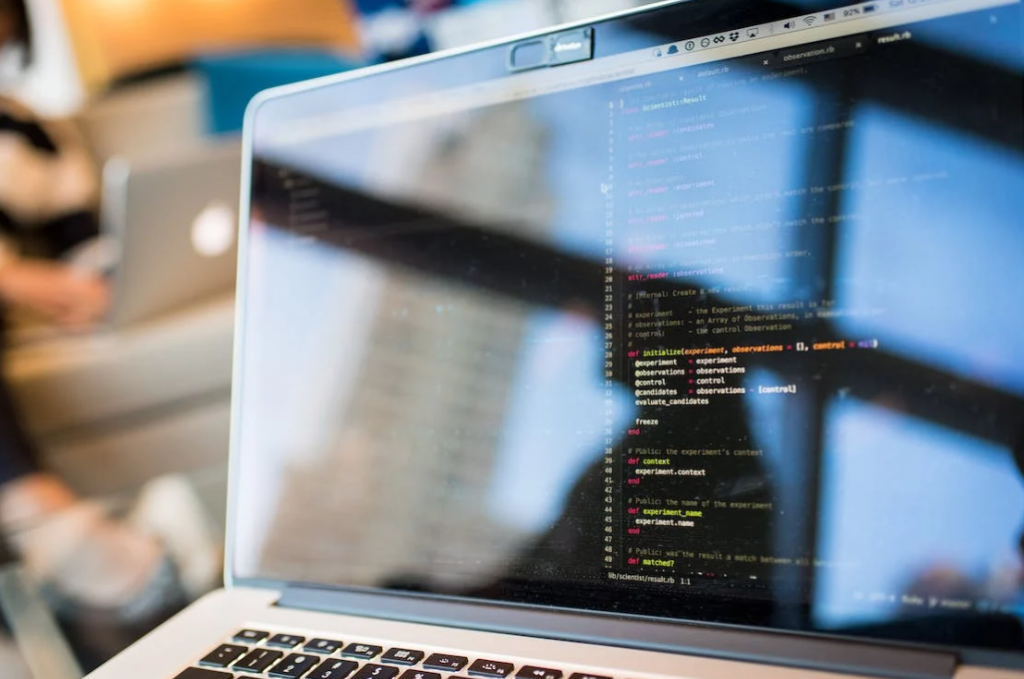
The encodeURI() function encodes all characters within the provided string, with the exception of A-Z, a-z, 0-9, semicolon (;), comma (,), forward slash (/), question mark (?), colon (:), at symbol (@), ampersand (&), equal sign (=), plus sign (+), dollar sign ($), hyphen (-), underscore (_), period (.), exclamation mark (!), tilde (~), asterisk (*), single quotation mark (‘), parentheses (), and hash (#). It is the recommended choice when the string you are encoding represents a complete URL.
const partOfURL = 'my-page#with,speci@l&/"characters"?';
const fullURL = 'https://my-website.com/my-page?query="a%b"&user=1';
encodeURI(partOfURL); // Bad, does not escape all special characters
// 'my-page#with,speci@l&/%22characters%22?'
encodeURI(fullURL); // Good, encoded URL is valid
// 'https://my-website.com/my-page?query=%22this%25thing%22&user=1'
Conclusion
JavaScript’s encodeURI() and encodeURIComponent() functions play crucial roles in web development by enabling the encoding of special characters within URLs. It’s essential to understand their distinctions to use them effectively. encodeURIComponent() is the preferred choice when encoding specific portions of a URL, leaving out only a select few safe characters, making it ideal for maintaining URL integrity. On the other hand, encodeURI() should be employed when encoding an entire URL, as it encompasses a broader set of characters, ensuring the URL remains valid. Mastering the nuances of these functions empowers developers to handle URL encoding with precision, contributing to the overall security and functionality of web applications.
JavaScript: Object to Map Transformation
JavaScript is a versatile programming language that provides developers with a wide range of tools for working with strings and manipulating data. When dealing with web development and handling URLs, you often need to encode and decode special characters to ensure data integrity and security. Two commonly used functions for this purpose are encodeURI() and …
Switching to Object Literals for Cleaner JavaScript Code
JavaScript is a versatile programming language that provides developers with a wide range of tools for working with strings and manipulating data. When dealing with web development and handling URLs, you often need to encode and decode special characters to ensure data integrity and security. Two commonly used functions for this purpose are encodeURI() and …