Exploring JavaScript’s Event Loop Mechanism
The Event Loop can be quite bewildering for many developers, yet it remains a crucial cornerstone in the realm of the JavaScript engine. It serves as the fundamental mechanism that enables JavaScript to operate as a single-threaded entity while also having the capability to execute tasks in a non-disruptive fashion. To attain a thorough understanding of the Event Loop, it becomes essential to explore the inner workings of the JavaScript engine, encompassing elements like the Call Stack, Tasks, Microtasks, and the respective Queues they inhabit. Join us as we embark on an expedition to dissect each of these components in-depth.
JavaScript’s Call Stack: A Comprehensive Overview
JavaScript, a versatile programming language renowned for its ability to create interactive and dynamic web applications, employs a unique and crucial data structure known as the Call Stack. This Call Stack, functioning on the Last In, First Out (LIFO) principle, plays a pivotal role in orchestrating the execution of functions within its runtime environment. To truly comprehend its significance, let’s take a deep dive into its inner workings.
The Call Stack: Unveiling the Mechanics
At its core, the Call Stack is a structured mechanism for managing the order of function executions. It acts as a vigilant supervisor, ensuring that each function completes its tasks in the right sequence. Here’s how it operates:
1. Initialization
– The Call Stack begins as an empty structure, waiting to be populated as the program unfolds.
2. Function Invocation
– As the program progresses, when a function like `foo()` is called, it is added to the Call Stack. This is akin to stacking a new task on top of the existing ones.
3. Execution and Deletion
– While `foo()` starts its execution, something interesting happens. Before it finishes its job, it’s removed or “popped” from the Call Stack. It’s important to note that this removal doesn’t mean the function is completed; it merely signifies the initiation of tasks within `foo()`.
4. Nested Functions
– Within the `foo()` function, if there are statements like `console.log(‘foo’)`, they are also placed on the Call Stack. They get pushed, executed (resulting in ‘foo’ being printed to the console), and then swiftly removed.
5. Subsequent Function Call
– Now, consider the function `bar()`, which is invoked from within `foo()`. Following the same protocol, `bar()` is stacked onto the Call Stack.
6. Completion of bar() Function
– As `bar()` begins execution, it encounters the `console.log(‘bar’)` statement. This statement, too, joins the Call Stack. It’s pushed, executed (‘bar’ appears on the console), and subsequently removed.
7. End State
– When all function invocations and their internal instructions conclude their execution, the Call Stack reverts to its original empty state, devoid of any entries.
Understanding Tasks and Their Respective Queue
Tasks are essentially predetermined, synchronized segments of code. During their execution, they commandeer the Call Stack, an essential component in managing how functions run. Furthermore, tasks have the capability to arrange the initiation of other tasks. What’s notable is that in intervals between tasks, the browser is free to update its visual rendering.
Where do these tasks find their temporary residence prior to their execution? Welcome to the Task Queue, a specialized storage mechanism functioning on the principle of First In, First Out (FIFO). This meticulous arrangement guarantees that the task occupying the forefront of the queue will be the inaugural one to undergo execution. An archetypal illustration of such a task encompasses the callback function, which springs into action in response to a precise event, like the moment an event listener identifies a specific occurrence. Another illustration lies in the callback summoned forth through the agency of the setTimeout() function.
Deciphering Microtasks and Their Queue
Microtasks share many similarities with tasks: they’re predetermined, synchronized segments of code, and they too, gain exclusive rights to the Call Stack when they’re running. However, there’s a key distinction. After every task wraps up its execution, and before any new rendering occurs, the Microtask Queue must be completely emptied.
This Microtask Queue, like its counterpart for tasks, also follows the FIFO principle. Examples of microtasks that might be familiar include callbacks of Promises and those associated with MutationObserver.
It’s worth noting that there’s an alternate terminology for Microtasks and the Microtask Queue: they’re sometimes labeled as Jobs and the Job Queue respectively.
The Dynamics of the Event Loop
At the heart of this intricate system lies the Event Loop. It’s a ceaseless loop with one primary objective: continuously verifying if the Call Stack is vacant. Once confirmed, the Event Loop is responsible for managing the placement and execution of tasks and microtasks on the Call Stack, and it plays an integral role in the rendering procedure.
This loop is orchestrated through four pivotal steps:
- Script Evaluation: The initial phase involves the direct and synchronized execution of scripts. This continues relentlessly until the Call Stack is devoid of any tasks;
- Task Orchestration: The Event Loop zeroes in on the premier task waiting in the Task Queue and engages it. This continues until, once again, the Call Stack is empty;
- Microtask Regulation: Transitioning its focus to the Microtask Queue, the Event Loop targets the leading microtask. It ensures its execution and then repeatedly processes subsequent microtasks in the queue until it’s empty;
- Rendering Process: The UI is refreshed, visual updates take place, and the loop returns to the second phase, thereby maintaining its continuous cycle.
This intricate interplay between tasks, microtasks, and the Event Loop ensures seamless functionality and user experience in various applications.
Event Loop Through a Detailed Example
In delving deep into the Event Loop mechanism, it’s advantageous to examine a thorough real-world example. By doing this, the concepts surrounding the Event Loop can be better grasped:
console.log('Script start');
setTimeout(() => console.log('setTimeout()'), 0);
Promise.resolve()
.then(() => console.log('Promise.then() #1'))
.then(() => console.log('Promise.then() #2'));
console.log('Script end');
Upon execution, the following order of logs is observed:
Script start
Script end
Promise.then() #1
Promise.then() #2
setTimeout()
For many, this sequence might appear perplexing. To shed light on the process, a comprehensive breakdown of the sequence is presented:
Introduction to JavaScript Execution Mechanics:
The JavaScript language operates in an intricately organized manner, ensuring smooth and efficient code execution. To fully grasp this, a detailed look at the system’s sequential steps is indispensable. A deep dive into this procedure provides an illuminating understanding of how scripts come to life, run, and culminate.
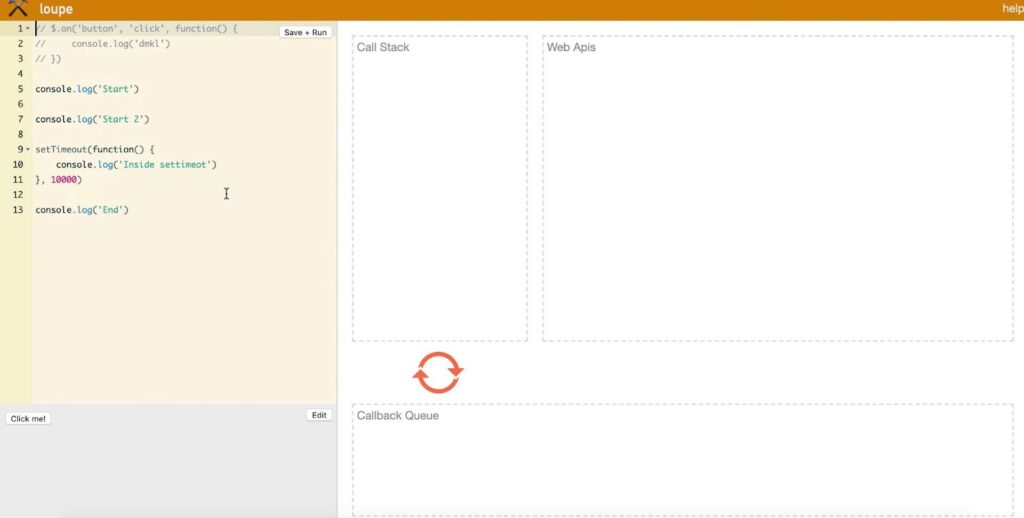
1. The Kick-off:
Upon the commencement of a JavaScript script, the Call Stack, a foundational mechanism responsible for managing function calls, finds itself empty and prepared for action. At this moment, the Event Loop, which is the heartbeat of JavaScript’s asynchronous nature, starts its mission by evaluating the given script.
2. Highlighting the Start:
The moment console.log() enters the scene, it is placed onto the Call Stack. Without delay, this function runs and displays the message indicating the beginning of the script, which reads ‘Script start’.
3. Introducing a Timeout:
Following that, setTimeout() is introduced onto the Call Stack. As this function executes, it creates a new Task dedicated to its callback function. This Task then assumes a waiting position in the Task Queue, anticipating its turn for execution.
4. Diving into Promises:
Shortly after, Promise.prototype.resolve() is ushered onto the Call Stack. This move seamlessly segues into the next action where Promise.prototype.then() is invoked.
5. Microtask Management:
Upon processing Promise.prototype.then(), an innovative Microtask is born for its associated callback function. This Microtask then comfortably positions itself in the specially designated Microtask Queue.
6. Signaling the End:
Yet another console.log() method is swiftly lifted onto the Call Stack, effectively marking the script’s preliminary conclusion by broadcasting the message ‘Script end’.
7. Microtask Execution:
With the primary script assessment finalized, the Event Loop’s attention shifts, concentrating on processing the Microtask, specifically the one associated with Promise.prototype.then(), queued previously.
8. Activating the First Promise Callback:
The familiar console.log() function is ushered onto the Call Stack, this time relaying the message ‘Promise.then() #1’.
9. Preparing a Second Promise Callback:
Promise.prototype.then() re-emerges onto the Call Stack, creating an additional queue entry for its callback function, which now anticipates its execution in the Microtask Queue.
10. Scrutinizing the Microtask Queue:
The ever-attentive Event Loop examines the Microtask Queue. On discovering unexecuted tasks, it swiftly processes the recently queued Microtask related to Promise.prototype.then().
11. Running the Second Promise Callback:
Without missing a beat, the console.log() function re-enters the Call Stack, revealing the message ‘Promise.then() #2’.
12. Potential Visual Updates:
If there arise any necessary adjustments, the system might proceed with a re-rendering operation, ensuring the visual elements reflect the latest changes.
13. Task Queue Engagement:
With the Microtask Queue now cleared, the Event Loop pivots its attention to the Task Queue, gearing up to activate the first Task linked with setTimeout() created earlier.
14. Realizing the setTimeout Callback:
In its conclusive act, the console.log() function gracefully returns to the Call Stack, this time announcing the message ‘setTimeout()’.
15. Possible Re-rendering Phase:
Once more, there’s a window of opportunity for re-rendering. Should there be any critical visual updates, the system will engage in another rendering cycle.
16. The Grand Finale:
As the journey concludes, the script wraps up its tasks, leaving the Call Stack in its pristine, unoccupied state. This marks the termination of this intricate process.
By delving into this elaborate procedure, developers can unlock profound knowledge about JavaScript’s inner workings. Such comprehension not only fuels curiosity but is also invaluable when refining code quality, enhancing performance, and troubleshooting potential challenges.
Notes
- The Event Loop’s script evaluation phase is akin to handling a Task on its own accord. When employing setTimeout(), it’s crucial to note that its second argument denotes a minimal timeframe for execution, rather than an assured one. This subtlety arises from the strict sequential execution of Tasks, with the possibility of Microtasks interleaving;
- Comparatively, the behavior of the event loop in Node.js mirrors the concept but bears a few distinctions. Chief among these disparities is the absence of a rendering phase;
- In times of yore, older browser iterations exhibited a certain disregard for the prescribed order of operations, leading to potential variations in the execution sequence of Tasks and Microtasks.
Conclusion
The pivotal role of the Event Loop lies in the execution of JavaScript code. It commences its duty by meticulously assessing and executing the script, subsequently handling Tasks and Microtasks with finesse.
Tasks and Microtasks, these meticulously scheduled, synchronous blocks of code, are treated with utmost precision. Each one is given its moment in the spotlight, being arranged diligently in either the Task Queue or the Microtask Queue, depending on their nature.
To orchestrate this intricate dance, the Call Stack gracefully steps in to monitor the flow of function calls.
Whenever the time arrives for Microtasks to shine, it is imperative that the Microtask Queue is emptied to create a clean slate for the next Task to step into the limelight.
Rendering, on the other hand, gracefully unfolds its spectacle between Tasks, finding its rightful place but gracefully abstaining from interfering between Microtasks.
Unlocking the Power of JavaScript’s Lowercasing Features
The Event Loop can be quite bewildering for many developers, yet it remains a crucial cornerstone in the realm of the JavaScript engine. It serves as the fundamental mechanism that enables JavaScript to operate as a single-threaded entity while also having the capability to execute tasks in a non-disruptive fashion. To attain a thorough understanding …
JavaScript DateDiff: A Comprehensive Guide
The Event Loop can be quite bewildering for many developers, yet it remains a crucial cornerstone in the realm of the JavaScript engine. It serves as the fundamental mechanism that enables JavaScript to operate as a single-threaded entity while also having the capability to execute tasks in a non-disruptive fashion. To attain a thorough understanding …