Comprehensive Guide: Initializing JavaScript Arrays
Initializing arrays in JavaScript is a fundamental task, and there are various techniques to choose from, each with its own set of considerations. In this article, we explore the different methods for initializing arrays, their advantages, and when to use them effectively.
Array() Constructor
The most straightforward way to create an array is by using the Array() constructor. This method allows you to specify the desired length of the array. However, it has some limitations, especially when it comes to handling empty values or “holes” in the array.
const arr = Array(3); // [ , , ] – 3 empty slotsarr.map(() => 1); // [ , , ] – map() skips empty slotsarr.map((_, i) => i); // [ , , ] – map() skips empty slotsarr[0]; // undefined – actually, it is an empty slot |
The Array() constructor is not the most versatile option, and it’s often better suited for creating arrays with specified values rather than dealing with dynamic initialization.
Array.from()
Array. from() is a versatile method for creating arrays from array-like or iterable objects. It excels at converting array-like objects (e.g., arguments, NodeList) or iterables (e.g., Set, Map, Generator) into actual arrays. You can also use it to create an array of a specific length by passing an object with a length property. This method allows you to pass a mapping function as a second argument, which is handy for initializing arrays with specific values.
const arr = Array.from({ length: 3 }); // [undefined, undefined, undefined]arr.map(() => 1); // [1, 1, 1]arr.map((_, i) => i); // [0, 1, 2]const staticArr = Array.from({ length: 3 }, () => 1); // [1, 1, 1]const indexArr = Array.from({ length: 3 }, (_, i) => i); // [0, 1, 2] |
Array.from() provides flexibility and works well when you need to create arrays from other iterable data structures or when you require more control over the array’s initialization.
Array.prototype.fill()
When you need to fill an existing array with a specific value, Array.prototype.fill() is a convenient choice. This method allows you to populate an array with the same value efficiently.
const nullArr = new Array(3).fill(null); // [null, null, null]const staticArr = Array.from({ length: 3 }).fill(1); // [1, 1, 1]const indexArr = Array(3).fill(null).map((_, i) => i); // [0, 1, 2] |
Using Array.prototype.fill() is particularly efficient when combined with the Array() constructor, as it allows you to avoid empty slots in the resulting array.
Array.prototype.map()
While Array.from() supports a mapping function via a second argument, some developers find it hard to read. In such cases, or when you need access to the array itself during mapping, Array.prototype.map() is a viable option. However, keep in mind that it does not handle empty values well.
const arr = Array(3).map(() => 1); // [ , , ] – map() skips empty slotsconst staticArr = Array.from({ length: 3 }).map(() => 1); // [1, 1, 1]const indexArr = Array.from({ length: 3 }).map((_, i) => i); // [0, 1, 2]const fractionArr = Array.from({ length: 3 }).map((_, i, a) => i / a.length); // [0, 0.5, 1] |
Array.prototype.map() offers flexibility and readability, especially when dealing with non-empty arrays.
A Note on Performance
In most cases, the performance of these array initialization methods is not a significant concern. The Array() constructor tends to be the fastest. Interestingly, even when chaining an Array.prototype.map() call after the constructor to create dynamic values, it can still be the best option for initializing an array with a single value.
const initializeArrayWithValues = (n, val = 0) => Array(n).fill(val);const initializeMappedArray = (n, mapFn = (_, i) => i) => Array(n).fill(null).map(mapFn);initializeArrayWithValues(4, 2); // [2, 2, 2, 2]initializeMappedArray(4, (_, i) => i * 2); // [0, 2, 4, 6] |
Comparison Table
Method | Description | Pros | Cons |
---|---|---|---|
Array() constructor | Creates an array with empty slots and limitations on populating values. | Simple to use | Generates arrays with holes and undefined values. |
Array.from() | Converts array-like or iterable objects into arrays and allows mapping during initialization. | Versatile and flexible | Slightly slower than the Array() constructor. |
Array.prototype.fill() | Fills an existing array with the same value, useful for initializing arrays with a single value. | Efficient when filling with a value | Limited in its ability to populate dynamic values. |
Array.prototype.map() | Provides flexibility and readability for initialization, especially when dynamic values are needed. | Readable and flexible | Doesn’t work well with empty values; can be slower. |
This table compares the four methods of initializing JavaScript arrays discussed in the article, highlighting their pros and cons.
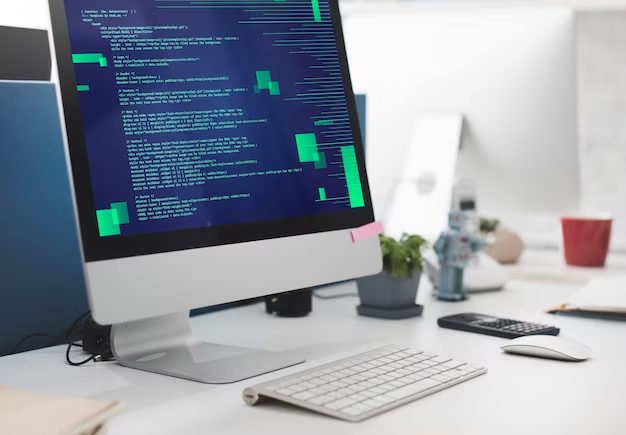
Conclusion
In JavaScript, initializing arrays is a common task, and choosing the right method can impact performance and readability. While each method has its advantages and disadvantages, understanding when and how to use them can help you write more efficient and maintainable code. Consider the specific requirements of your project to determine the best approach for array initialization.
FAQ
The Array() constructor is generally the fastest, especially when combined with Array.prototype.fill() for initializing with a single value.
Use Array.from() when you need to convert array-like or iterable objects into arrays or when you want to apply a mapping function during initialization.
Yes, Array.prototype.map() provides flexibility for initializing arrays with dynamic values and can be a readable option.
You can use Array.from() with a mapping function or Array.prototype.map() to populate values and avoid empty slots.
Array.from() is the most versatile method, as it can handle various input types and allows mapping during initialization.
JavaScript Naming Conventions: Step-by-Step Guide
Initializing arrays in JavaScript is a fundamental task, and there are various techniques to choose from, each with its own set of considerations. In this article, we explore the different methods for initializing arrays, their advantages, and when to use them effectively. Array() Constructor The most straightforward way to create an array is by using …
JavaScript Page Reloading: Methods and Best Practices
Initializing arrays in JavaScript is a fundamental task, and there are various techniques to choose from, each with its own set of considerations. In this article, we explore the different methods for initializing arrays, their advantages, and when to use them effectively. Array() Constructor The most straightforward way to create an array is by using …