How to Copy Text to Clipboard in JavaScript
Copying text to the clipboard is a common requirement when developing websites or web applications. It allows users to easily share content with a single click. In this guide, we will explore various techniques to copy text to the clipboard using JavaScript. We’ll also discuss best practices and compatibility considerations.
Introduction to Clipboard API
If your goal is to support modern browsers, the asynchronous Clipboard API is the recommended approach. It is widely supported in modern browsers and provides a straightforward and secure way to update the clipboard’s contents.
To copy text to the clipboard using the Clipboard API, follow these steps:
const copyToClipboard = (str) => { if (navigator && navigator.clipboard && navigator.clipboard.writeText) { return navigator.clipboard.writeText(str); } return Promise.reject(‘The Clipboard API is not available.’);}; |
This code checks if the required clipboard functions are available and then uses navigator.clipboard.writeText(str) to copy the provided text to the clipboard. If the Clipboard API is not available, it returns a rejected promise.
Fallback Option: Document.execCommand(‘copy’)
While the Clipboard API is well-supported in modern browsers, you may encounter situations where you need to support older browsers. In such cases, you can use Document.execCommand(‘copy’) as a fallback option.
Here are the steps to copy text to the clipboard using this method:
- Create a <textarea> element and set its value to the text you want to copy;
- Append the <textarea> element to the document, but hide it using CSS to prevent any visual disturbance;
- Select the contents of the <textarea> element using HTMLInputElement.select();
- Use Document.execCommand(‘copy’) to copy the contents of the <textarea> to the clipboard;
- Remove the <textarea> element from the document.
Here’s an example implementation:
const copyToClipboard = (str) => { const el = document.createElement(‘textarea’); el.value = str; el.setAttribute(‘readonly’, ”); el.style.position = ‘absolute’; el.style.left = ‘-9999px’; document.body.appendChild(el); el.select(); document.execCommand(‘copy’); document.body.removeChild(el);}; |
Please note that this method only works as a result of a user action, such as inside a click event listener, due to the way Document.execCommand() operates.
Best Practices and Considerations
- Error Handling: When using the Clipboard API, consider handling any potential errors gracefully to provide a good user experience;
- User Feedback: After copying text to the clipboard, consider providing visual feedback to the user to confirm the action’s success;
- Compatibility: Determine your target audience and browser support requirements when choosing between the Clipboard API and Document.execCommand(‘copy’);
- Security: Be cautious when dealing with clipboard interactions, as they can be perceived as a security risk. Only copy relevant and user-initiated content to the clipboard.
Comparison Table
Here’s a comparative table that summarizes the key differences between using the Clipboard API and Document.execCommand(‘copy’) to copy text to the clipboard in JavaScript:
Feature | Clipboard API | Document.execCommand(‘copy’) |
---|---|---|
Browser Compatibility | Supported in modern browsers | Supported in older browsers as a fallback |
Simplicity | Requires fewer steps and is more intuitive | Involves multiple steps and manual element creation |
Error Handling | Provides promise-based error handling | Limited error handling capabilities |
User Interaction Required | No user interaction required | Typically requires user interaction (e.g., button click) |
Visual Feedback | Easily implementable with feedback to the user | Custom implementation required for feedback |
Security Considerations | Generally considered more secure | May raise security concerns, especially in older browsers |
Use Cases | Recommended for modern web applications | Suitable for projects with legacy browser support |
This table offers a quick overview of the main distinctions between the two methods, helping you choose the one that best suits your project’s needs. Remember to consider factors like browser compatibility, user experience, and security when deciding which approach to implement.
Clipboard Management Best Practices
Effective clipboard management is crucial for a seamless user experience on your website. Here are some best practices to consider:
- User Feedback: Always provide clear feedback to the user when they attempt to copy text to the clipboard. A simple success message or notification can enhance user satisfaction;
- Cross-Browser Testing: Test your clipboard copy functionality across various browsers to ensure compatibility. While the Clipboard API is preferred for modern browsers, having a fallback method like Document.execCommand(‘copy’) can improve usability for older browser users;
- Security Measures: Be cautious when dealing with sensitive information on the clipboard. Avoid automatically copying sensitive data, and always seek user consent when handling data like passwords or personal information;
- Accessibility: Ensure that your clipboard copy feature is accessible to all users, including those with disabilities. Use ARIA attributes and provide keyboard shortcuts when possible;
- Error Handling: Implement error handling mechanisms, especially when using the Clipboard API. Inform users when copying fails and provide alternative methods or instructions.
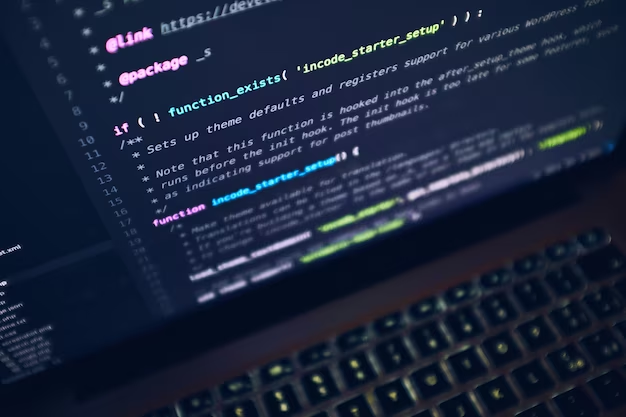
Conclusion
In this comprehensive guide, we’ve explored the various methods of copying text to the clipboard using JavaScript. Whether you prefer the modern and asynchronous Clipboard API for modern browsers or the fallback method with Document.execCommand(‘copy’) for older browsers, you now have the tools to implement this essential functionality on your website.
Remember to consider user feedback, cross-browser compatibility, security measures, accessibility, and error handling when implementing clipboard copy features. By doing so, you can enhance the user experience and ensure a seamless interaction with your website.
Now, let’s address some frequently asked questions to clarify any lingering doubts.
FAQ
While the Clipboard API is supported in most modern browsers, it may not work in older or less common browsers. To ensure wider compatibility, consider using a fallback method like Document.execCommand(‘copy’) for older browser support.
You can display a success message or notification on your website when the copy action is successful. This feedback informs users that their desired content has been copied to the clipboard.
Yes, when handling sensitive information, such as passwords or personal data, it’s essential to seek user consent and handle data securely. Avoid automatic copying of sensitive data and implement robust security measures.
Implement proper error handling to inform users when copying fails. You can provide alternative methods or instructions for users to copy the content manually.
To make your clipboard copy feature accessible, use ARIA attributes to enhance screen reader compatibility. Additionally, provide keyboard shortcuts and ensure that the feature is operable using a keyboard.
JavaScript Page Reloading: Methods and Best Practices
Copying text to the clipboard is a common requirement when developing websites or web applications. It allows users to easily share content with a single click. In this guide, we will explore various techniques to copy text to the clipboard using JavaScript. We’ll also discuss best practices and compatibility considerations. Introduction to Clipboard API If …
Adding Key-Value Pairs to JavaScript Objects
Copying text to the clipboard is a common requirement when developing websites or web applications. It allows users to easily share content with a single click. In this guide, we will explore various techniques to copy text to the clipboard using JavaScript. We’ll also discuss best practices and compatibility considerations. Introduction to Clipboard API If …