Adding Key-Value Pairs to JavaScript Objects
Are you looking to add key-value pairs to a JavaScript object? This is a common task in web development, and there are multiple ways to achieve it. In this guide, we’ll explore various approaches, their differences, and when to use each method.
Dot Notation
const obj = { a: 1 };obj.b = 2;obj.c = 3;// obj = { a: 1, b: 2, c: 3 } |
Dot notation is the most straightforward way to add a key-value pair to an object. It’s commonly used and suitable for most situations.
Square Bracket Notation
const obj = { a: 1 };const bKey = ‘b’;obj[bKey] = 2;obj[‘c’] = 3;// obj = { a: 1, b: 2, c: 3 } |
Square bracket notation is useful when dealing with dynamic keys but can also work with static keys. It functions similarly to dot notation.
Object.assign()
const obj = { a: 1 };Object.assign(obj, { b: 2 }, { c: 3 });// obj = { a: 1, b: 2, c: 3 } |
Object. assign() allows you to add multiple properties to an object at once, and can also merge two or more objects. It’s less performant but offers flexibility.
Object.defineProperty()
const obj = { a: 1 };Object.defineProperty(obj, ‘b’, { value: 2, enumerable: true, configurable: true, writable: true});Object.defineProperty(obj, ‘c’, { value: 3, enumerable: true, configurable: true, writable: true});// obj = { a: 1, b: 2, c: 3 } |
Object.defineProperty() is a highly performant way to add key-value pairs and allows precise property definition. It can also add multiple properties using Object.defineProperties().
Object Spread Operator
const obj = { a: 1 };const newObj = { …obj, b: 2, c: 3 };// obj = { a: 1 }// newObj = { a: 1, b: 2, c: 3 } |
The object spread operator creates a new object with added properties without mutating the original object. However, it’s less performant due to object creation.
Each method has its pros and cons, so choose the one that best suits your specific use case. Dot notation and square bracket notation are commonly used, while Object.assign() and Object.defineProperty() offer additional features. The object spread operator is convenient for creating new objects with added properties.
Considerations when Adding Properties
When adding key-value pairs to JavaScript objects, it’s essential to consider the specific requirements of your project. Here are some considerations:
- Performance: Depending on the method you choose, the performance of your code may vary. If performance is critical, consider using methods like dot notation or square bracket notation;
- Object Immutability: Some methods, such as the object spread operator, create a new object with added properties while leaving the original object unchanged. If you need to maintain the immutability of the original object, this approach might be suitable;
- Compatibility: Check the compatibility of your chosen method with the browsers or environments you intend to support. Some older browsers may not fully support newer techniques, so choose methods accordingly;
- Readability and Maintenance: Code readability and maintainability are crucial aspects of software development. Choose an approach that aligns with your team’s coding standards and makes the code easy to understand and maintain;
- Error Handling: Consider how each method handles errors or edge cases. Some methods may offer better error handling and reporting than others.
By taking these considerations into account, you can make an informed decision when adding key-value pairs to your JavaScript objects.
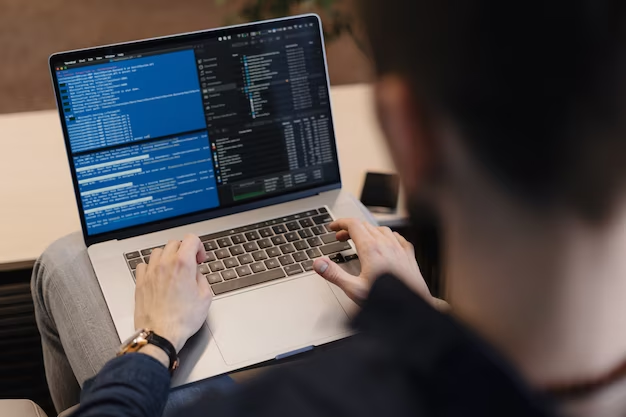
Conclusion
In the world of JavaScript, adding key-value pairs to objects is a fundamental task that every developer encounters. While there are multiple ways to accomplish this task, each method has its strengths and weaknesses.
Dot notation and square bracket notation are the most common and straightforward methods. They work well for static keys and offer good performance.
- Object.assign() is a versatile method for adding multiple properties at once or merging objects. It’s efficient but may not be necessary in all situations;
- Object.defineProperty() provides fine-grained control over property attributes but is less commonly used due to its complexity;
- Object spread operator is convenient for creating new objects with added properties while keeping the original object intact. However, it may have performance implications.
When deciding which method to use, consider factors like performance, object immutability, compatibility, readability, and error handling. The right choice depends on your specific use case and project requirements.
FAQ
The fastest method for adding properties to an object is usually dot notation or square bracket notation. These methods provide good performance and are suitable for most use cases.
Object. assign() is handy when you need to add multiple properties at once or merge objects. It’s a versatile method, but may not be necessary for simple property additions.
Object.defineProperty() allows fine-grained control over property attributes, such as configurability and writability. It’s useful when you need precise control over property behavior.
The object spread operator is convenient for creating new objects with added properties while keeping the original object intact. However, it may have performance implications, so use it judiciously.
Compatibility depends on the JavaScript environment you’re targeting. Newer methods may not be fully supported in older browsers, so consider your project’s compatibility requirements when choosing a method.
How to Copy Text to Clipboard in JavaScript
Are you looking to add key-value pairs to a JavaScript object? This is a common task in web development, and there are multiple ways to achieve it. In this guide, we’ll explore various approaches, their differences, and when to use each method. Dot Notation const obj = { a: 1 };obj.b = 2;obj.c = …
Manipulating URLs with JavaScript: A Comprehensive Guide
Are you looking to add key-value pairs to a JavaScript object? This is a common task in web development, and there are multiple ways to achieve it. In this guide, we’ll explore various approaches, their differences, and when to use each method. Dot Notation const obj = { a: 1 };obj.b = 2;obj.c = …