Enhance JSON Object Formatting with JavaScript
JSON (JavaScript Object Notation) is a widely used data interchange format due to its simplicity and ease of use. When working with JSON data in JavaScript, you may often come across situations where you need to format or “pretty-print” the JSON for better readability. Pretty-printing JSON makes it easier to debug, understand, and work with, especially when dealing with large or complex data structures.
In this article, the focus will be on exploring various methods for pretty-printing a JSON object using JavaScript.
What is Pretty-Printing?
Pretty-printing, in the context of JSON, involves adding indentation to the data to improve its human readability. Achieving this is quite straightforward by employing the JSON.stringify() method with the appropriate parameters.
Here’s an example of non-pretty-printed JSON:
const obj = {
id: 1182,
username: 'johnsmith',
active: true,
emails: ['johnsmith@mysite.com', 'contact@johnsmi.th'],
};
JSON.stringify(obj, null, 2);
// {
// "id": 1182,
// "username": "johnsmith",
// "active": true,
// "emails": [
// "johnsmith@mysite.com"
// "contact@johnsmi.th"
// ]
// }
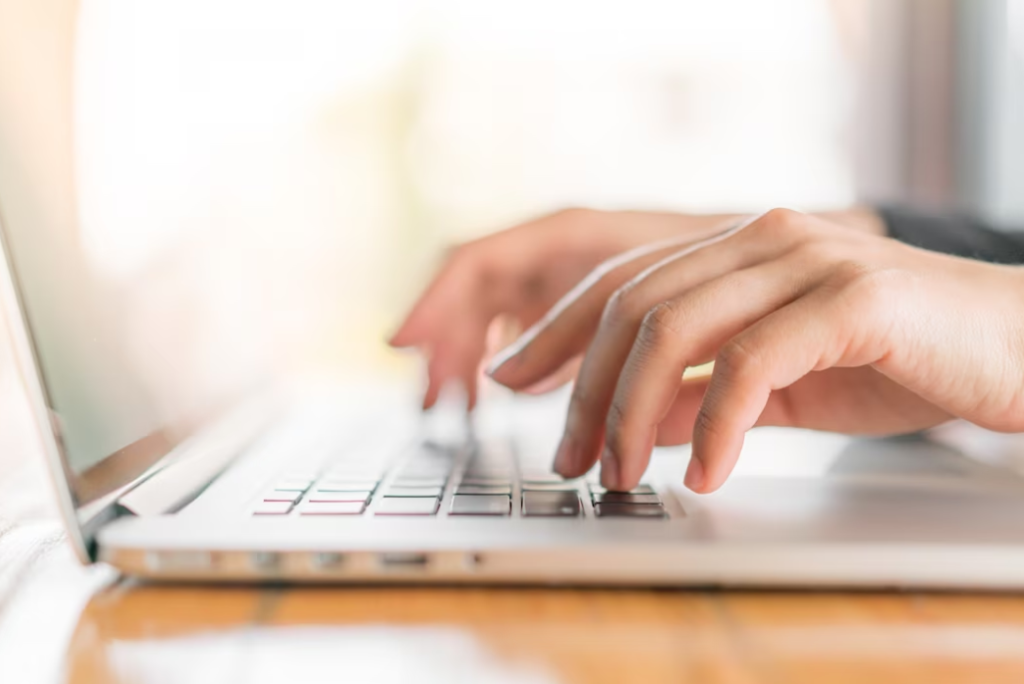
As demonstrated in this example, the third parameter of JSON.stringify() dictates the number of spaces to use for indenting each level within the object. Moreover, you have the option to utilize the second parameter to define a replacer function. This feature proves useful when you wish to apply custom formatting to particular value types or specific key-value pairs.
const obj = {
id: 1182,
username: 'johnsmith',
active: true,
emails: ['johnsmith@mysite.com', 'contact@johnsmi.th'],
};
const replacer = (key, value) => {
if (key === 'id') return value.toString(16);
if (key === 'username') return `@${value}`;
if (key === 'emails') return `${value[0]} +${value.length - 1} more`;
return value;
};
JSON.stringify(obj, replacer, 2);
// {
// "id": "0x4e2",
// "username": "@johnsmith",
// "active": true,
// "emails": "johnsmith@mysite.com +1 more"
// }
Conclusion
Pretty-printing JSON in JavaScript is a straightforward task, thanks to the built-in JSON.stringify() method. By using the replacer and space parameters, you can easily format JSON data to improve its readability. If you require more advanced formatting options, consider using external libraries like json-beautify or prettyjson.
Formatting JSON not only enhances readability but also makes it easier to work with JSON data during development, debugging, and sharing with others. So, whether you’re dealing with configuration files, API responses, or any other JSON data, pretty-printing is a valuable technique to have in your JavaScript toolkit.
Switching to Object Literals for Cleaner JavaScript Code
JSON (JavaScript Object Notation) is a widely used data interchange format due to its simplicity and ease of use. When working with JSON data in JavaScript, you may often come across situations where you need to format or “pretty-print” the JSON for better readability. Pretty-printing JSON makes it easier to debug, understand, and work with, …
JS Debounce: How to Delay a Function in JavaScript
JSON (JavaScript Object Notation) is a widely used data interchange format due to its simplicity and ease of use. When working with JSON data in JavaScript, you may often come across situations where you need to format or “pretty-print” the JSON for better readability. Pretty-printing JSON makes it easier to debug, understand, and work with, …