Linked List in JavaScript: Explanation
A linked list is a fundamental data structure in computer science that consists of a collection of elements, each of which points to the next one. In this article, we’ll explore the concept of a linked list in JavaScript, including its properties, operations, and implementation.
Definition
In a linked list, each element, known as a node, comprises two main properties:
- value: The value of the node;
- next: A reference to the next node in the list. It’s null if there is no next node, indicating the end of the list.
The linked list has three essential properties:
- size: The total number of elements in the list;
- head: The first element (node) in the list;
- tail: The last element (node) in the list.
Main Operations
Linked lists support several fundamental operations:
- insertAt: Inserts an element at a specific index;
- removeAt: Removes the element at a specific index;
- getAt: Retrieves the element at a specific index;
- clear: Empties the linked list;
- reverse: Reverses the order of elements in the linked list.
Implementation
Here’s an example implementation of a linked list in JavaScript:
class LinkedList { constructor() { this.nodes = []; } get size() { return this.nodes.length; } get head() { return this.size ? this.nodes[0] : null; } get tail() { return this.size ? this.nodes[this.size – 1] : null; } insertAt(index, value) { const previousNode = this.nodes[index – 1] || null; const nextNode = this.nodes[index] || null; const node = { value, next: nextNode }; if (previousNode) previousNode.next = node; this.nodes.splice(index, 0, node); } // Other methods: insertFirst, insertLast, getAt, removeAt, clear, reverse, iterator} |
This LinkedList class allows you to create and manipulate linked lists in JavaScript. It provides methods for inserting, removing, and manipulating elements in the list.
Example Usage
const list = new LinkedList(); list.insertFirst(1);list.insertFirst(2);list.insertFirst(3);list.insertLast(4);list.insertAt(3, 5); console.log(list.size); // 5console.log(list.head.value); // 3console.log(list.tail.value); // 4console.log([…list.map(e => e.value)]); // [3, 2, 1, 5, 4] list.removeAt(1);console.log(list.getAt(1).value); // 1console.log([…list.map(e => e.value)]); // [3, 1, 5, 4] list.reverse();console.log([…list.map(e => e.value)]); // [4, 5, 1, 3] list.clear();console.log(list.size); // 0 |
Comparison Table: Arrays vs. Linked Lists
Feature | Arrays | Linked Lists |
---|---|---|
Storage Efficiency | Excellent | Efficient for insertion |
Insertion/Deletion Speed | Slower for shifting | Faster for mid-list ops |
Random Access | O(1) | O(n) |
Size Manipulation | Cumbersome | Easy |
Memory Usage | Contiguous allocation | Dynamic allocation |
Explore the differences between arrays and linked lists in terms of various features and functionalities.
Real-World Applications of Linked Lists
Linked lists are not just abstract data structures; they have real-world applications that make them essential in software development. Let’s explore some of these practical use cases:
1. Music Playlists
Imagine creating a music playlist in your favorite music app. Each song in the playlist is like a node in a linked list. You can easily move between songs by following the “next” pointer, and you can add or remove songs without much hassle. Linked lists simplify the management of playlists and ensure a smooth music playback experience.
2. Browser History
Your web browser’s history functionality is another example of linked lists in action. Each visited webpage is a node in the linked list. When you click the “back” or “forward” button, you’re essentially traversing this list. It allows you to navigate through your browsing history seamlessly.
3. Undo/Redo Functionality
Many software applications, such as text editors and graphic design tools, provide undo and redo features. Linked lists are often used to implement these functionalities. Each action (e.g., typing, drawing, or formatting) is recorded as a node in the linked list. When you undo or redo an action, you traverse the list accordingly.
4. Task Management
Task management applications use linked lists to handle tasks and to-do lists. Each task is represented as a node in the list, making it easy to add, complete, or delete tasks. Linked lists provide a flexible way to organize tasks and maintain their order.
5. Memory Management
In lower-level programming languages like C and C++, linked lists are used in memory management. The operating system maintains a linked list of free memory blocks, allowing efficient allocation and deallocation of memory for different processes.
6. Symbol Tables
Compilers and interpreters use symbol tables to keep track of variables, functions, and other identifiers in a program. Symbol tables often use hash tables or linked lists for efficient retrieval and management of symbols.
These real-world examples illustrate the versatility and practicality of linked lists in software development. Understanding how to implement and work with linked lists is essential for solving a wide range of programming challenges.
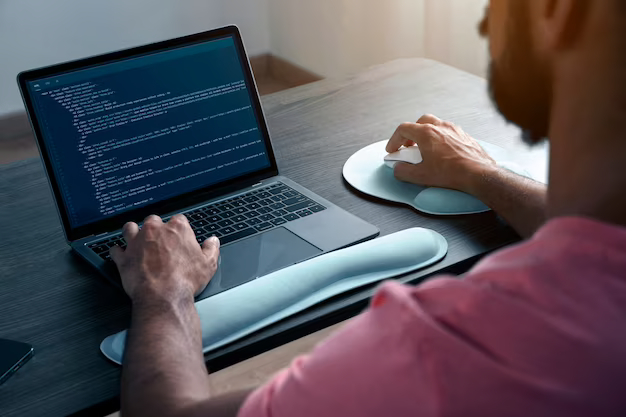
Conclusion
Linked lists are versatile data structures that find applications in various algorithms and scenarios. Understanding how to work with linked lists is fundamental for any JavaScript developer, as it forms the basis for more complex data structures and algorithms.
FAQ
1. What is a linked list?
A linked list is a linear data structure used in computer science to organize and store a collection of elements. Each element in a linked list is called a “node” and consists of two parts: the data or value, and a reference (or link) to the next node in the sequence.
2. What is the difference between an array and a linked list?
Arrays and linked lists are both used to store collections of data, but they differ in how they allocate and access memory. Arrays have a fixed size and store elements in contiguous memory locations, making random access fast. Linked lists, on the other hand, use dynamic memory allocation and provide efficient insertion and deletion operations, but they have slower random access times.
3. Can a linked list contain duplicate elements?
Yes, a linked list can contain duplicate elements. Each node in a linked list can hold a value, and duplicate values can exist in different nodes within the list.
4. How do I reverse a linked list?
To reverse a linked list, you can iterate through the list and update the “next” pointers of each node to reverse the order. There are iterative and recursive methods to achieve this.
Manipulating URLs with JavaScript: A Comprehensive Guide
A linked list is a fundamental data structure in computer science that consists of a collection of elements, each of which points to the next one. In this article, we’ll explore the concept of a linked list in JavaScript, including its properties, operations, and implementation. Definition In a linked list, each element, known as a …
JavaScript HTML Escape: A Crucial Skill for Web Security
A linked list is a fundamental data structure in computer science that consists of a collection of elements, each of which points to the next one. In this article, we’ll explore the concept of a linked list in JavaScript, including its properties, operations, and implementation. Definition In a linked list, each element, known as a …