Manipulating URLs with JavaScript: A Comprehensive Guide
In the ever-evolving world of web development, the ability to modify the URL without reloading the entire web page is a common requirement. JavaScript provides several methods to achieve this task, each with its own advantages and limitations. In this guide, we will explore the techniques for changing URLs in JavaScript, empowering you to enhance user experiences on your website.
Using the History API: The Modern Approach
History API is the preferred choice for modern web development. It not only allows you to change the URL seamlessly, but also offers additional functionalities. You have two options within the History API: history.pushState() and history.replaceState(). The choice between them depends on your specific needs.
history.pushState()
// Current URL: https://my-website.com/page_aconst nextURL = ‘https://my-website.com/page_b’;const nextTitle = ‘My new page title’;const nextState = { additionalInformation: ‘Updated the URL with JS’ }; // Create a new entry in the browser’s history, no page reloadingwindow.history.pushState(nextState, nextTitle, nextURL); |
history.replaceState()
// Current URL: https://my-website.com/page_aconst nextURL = ‘https://my-website.com/page_b’;const nextTitle = ‘My new page title’;const nextState = { additionalInformation: ‘Updated the URL with JS’ }; // Replace the current history entry, no page reloadingwindow.history.replaceState(nextState, nextTitle, nextURL); |
Both methods accept the same arguments, enabling you to provide a customized serializable state object as the first argument, specify a custom title (though most browsers may ignore it), and define the URL you want to add or replace in the browser’s history. Keep in mind that the History API restricts navigation to same-origin URLs, preventing you from navigating to entirely different websites.
Using the Location API: The Legacy Approach
While the Location API is considered less favorable for modern web development, it still serves a purpose, especially when dealing with legacy browsers. However, it comes with a significant drawback: it triggers a page reload. There are three ways to modify the URL using the Location API.
window. location.href
// Current URL: https://my-website.com/page_aconst nextURL = ‘https://my-website.com/page_b’; // Create a new entry in the browser’s history, followed by a page reloadwindow.location.href = nextURL; |
window.location.assign()
// Current URL: https://my-website.com/page_aconst nextURL = ‘https://my-website.com/page_b’; // Replace the current history entry, followed by a page reloadwindow. location.assign(nextURL); |
window.location.replace()
// Current URL: https://my-website.com/page_aconst nextURL = ‘https://my-website.com/page_b’; // Replace the current history entry, followed by a page reloadwindow.location.replace(nextURL); |
All three options in the Location API trigger a page reload, which may not be desirable in many cases. Unlike the History API, the Location API allows you to set the URL only, without additional arguments. Additionally, it doesn’t restrict navigation to same-origin URLs, potentially posing security risks if not used cautiously.
Comparison Table
Feature | History API | Location API |
---|---|---|
Page Reload | No page reload | Page reload |
Customizable State Object | Yes | No |
Customizable Title (limited support) | Yes | No |
Supports Same-Origin URLs | Yes | Potentially Security Concern |
Requires Same-Origin URLs | Yes | No |
Provides Additional Functionality | Yes | No |
This table should help you understand the key differences between these two methods for modifying URLs in JavaScript.
Advanced Techniques for URL Modification
While we’ve covered the fundamental methods for modifying URLs using JavaScript, there are advanced techniques you can explore to enhance your URL manipulation capabilities. Let’s delve into a few of these advanced options:
- URLSearchParams: The URLSearchParams API allows you to work with query parameters in URLs with ease. You can add, modify, or remove query parameters without reloading the page. This is especially useful when building interactive web applications that rely on dynamic URL parameters.
// Example: Add a query parameter to the current URLconst url = new URL(window.location.href);const params = new URLSearchParams(url.search);params.set(‘newParam’, ‘example’);url.search = params.toString();window.history.pushState({}, ”, url); |
- Hash Fragments: You can manipulate the URL’s hash fragment to create client-side routing within a single-page application. This technique enables you to change content on the page without triggering a full reload.
// Example: Change the hash fragment to navigate within a single page appwindow.location.hash = ‘#section2’; |
- Using Libraries: Many JavaScript libraries and frameworks, such as React Router and Vue Router, provide powerful routing capabilities. These libraries abstract much of the URL manipulation complexity and offer features like route matching, nested routes, and route guards.
- State Management: If you’re working with complex applications, consider integrating state management solutions like Redux (for React) or Vuex (for Vue.js). These libraries help manage application state and URL routing in a more structured way.
- Service Workers: For progressive web apps (PWAs), Service Workers can intercept and cache network requests, enabling offline functionality. This advanced technique allows you to control URL navigation even when the user is offline.
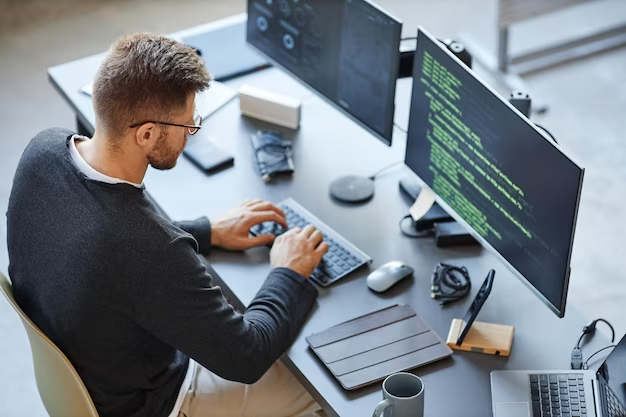
Changing URLs dynamically in JavaScript is a powerful feature that can greatly improve the user experience on your website. Whether you opt for the modern approach with the History API or the legacy approach using the Location API, understanding these methods empowers you to create more interactive and user-friendly web applications.
FAQ
The History API is a part of the HTML5 specification that allows JavaScript to interact with the browser’s history. It enables you to modify the URL displayed in the browser without triggering a full page reload. This is useful for creating smoother, more interactive web experiences.
Use history.pushState() when you want to add a new entry to the browser’s history stack. This means users can navigate back to the previous URL. Use history.replaceState() when you want to replace the current entry in the history stack. This can be helpful when you want to update the URL without creating a new history entry.
The History API only allows you to work with same-origin URLs. You cannot use it to navigate to entirely different websites. Additionally, while you can provide a custom title, many browsers may ignore it.
The Location API allows you to work with the current URL of the browser. It’s not as versatile as the History API because any modifications to the URL will trigger a page reload. It may be useful when dealing with legacy browsers or scenarios where a page reload is acceptable.
Yes, there can be security issues when using the Location API because it allows you to set URLs to different origins. This could potentially lead to security vulnerabilities if not handled carefully.
Adding Key-Value Pairs to JavaScript Objects
In the ever-evolving world of web development, the ability to modify the URL without reloading the entire web page is a common requirement. JavaScript provides several methods to achieve this task, each with its own advantages and limitations. In this guide, we will explore the techniques for changing URLs in JavaScript, empowering you to enhance …
Linked List in JavaScript: Explanation
In the ever-evolving world of web development, the ability to modify the URL without reloading the entire web page is a common requirement. JavaScript provides several methods to achieve this task, each with its own advantages and limitations. In this guide, we will explore the techniques for changing URLs in JavaScript, empowering you to enhance …