Comparing HTMLElement.innerText vs. Node.textContent
JavaScript offers two properties for accessing the textual content within an element: Node.textContent and HTMLElement.innerText. These properties may seem interchangeable, and indeed, many developers use them interchangeably without realizing the significant distinctions between the two.
Similarities
Examining the similarities between these two properties before delving into their differences can provide valuable insights. This approach can also offer clarity regarding their typical usage.
Consider an HTML element containing text:
<p id="greeting">Hi there! My name is <strong>Bubbles</strong>.</p>
Both these properties retrieve the element’s text content, encompassing any text from child elements while disregarding any HTML tags within the element’s content. Furthermore, they are capable of setting the element’s text content as well.
const greeting = document.getElementById('greeting');
greeting.innerText; // "Hi there! My name is Bubbles."
greeting.textContent; // "Hi there! My name is Bubbles."
greeting.innerText = 'Hello!'; // <p id="greeting">Hello!</p>
greeting.textContent = 'Hi!'; // <p id="greeting">Hi!</p>
Differences
Up until now, these two properties seem to perform the same task. Indeed, both come with handy features that greatly enhance their utility. However, variations between them become apparent when handling somewhat more intricate element content.
For instance, consider the following HTML element:
<div class="card">
<style>
p { color: red; }
strong { text-transform: uppercase; }
small { display: none; }
</style>
<p>Hi there!<br />My name is <strong>Bubbles</strong>.</p>
<small>And I'm a <strong>dog</strong>.</small>
</div>
Now, let’s examine the output of each of these two properties to observe their distinctions.
const card = document.querySelector('.card');
card.innerText;
/*
"Hi there!
My name is BUBBLES."
*/
card.textContent;
/*
"
p { color: red; }
strong { text-transform: uppercase; }
small { display: none; }
Hi there!My name is Bubbles.
And I'm a dog.
"
*/
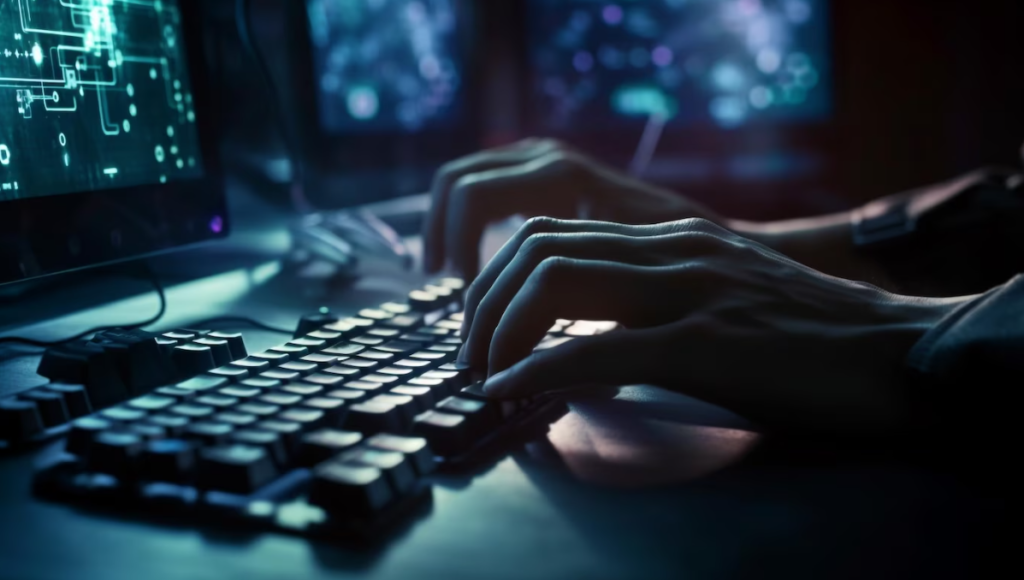
Considerably different in this scenario, isn’t it? HTMLElement.innerText aims to closely mimic what users observe in their browsers. Another way to conceptualize it is that its output should closely resemble the result a user would obtain if they were to select the element’s content and copy it to their clipboard.
- Hidden elements are disregarded according to this definition. This includes elements that don’t render, like <style> and <script>, as well as elements hidden through CSS. In the given example, the <small> element is concealed, so it doesn’t appear in the output of HTMLElement.innerText;
- HTMLElement.innerText produces normalized output. This implies that all whitespace is condensed into a single space, and line breaks are substituted with a single line break. If <br> tags are present, they are also acknowledged and replaced with line breaks;
- HTMLElement.innerText applies text transformations to the element’s content. In this instance, the <strong> element is altered to uppercase, resulting in HTMLElement.innerText reflecting this modification;
- Node.textContent retrieves the precise text content of the element, encompassing all whitespace and line breaks. However, it strips <br> tags without any replacement. Node.textContent also includes the text content of hidden elements, such as <style> and <script>, and doesn’t apply any text transformations.
Performance Considerations
Characteristic | HTMLElement.innerText | Node.textContent |
---|---|---|
Content Visualization | Approximates what’s visually rendered | Provides exact text content |
Excludes Hidden Elements | Ignores hidden elements (e.g., <style>, <script>) | Captures text content of all elements, including hidden ones |
Normalization | Normalizes whitespace and line breaks, respects <br> tags | Preserves all whitespace and line breaks, strips <br> tags |
Text Transformations | Applies text transformations from CSS | Does not apply text transformations |
Performance Considerations | Can trigger reflow for CSS calculations, potentially affecting performance | Efficient for plain text elements, reduces performance overhead |
However, it’s crucial to take into account an additional factor. While HTMLElement.innerText may seem like the intuitive choice, it does carry a performance consideration. This stems from the fact that it must consider the impact of CSS rules on rendering, potentially triggering a process known as “reflow.” Reflow can be computationally intensive, leading to unintended performance bottlenecks.
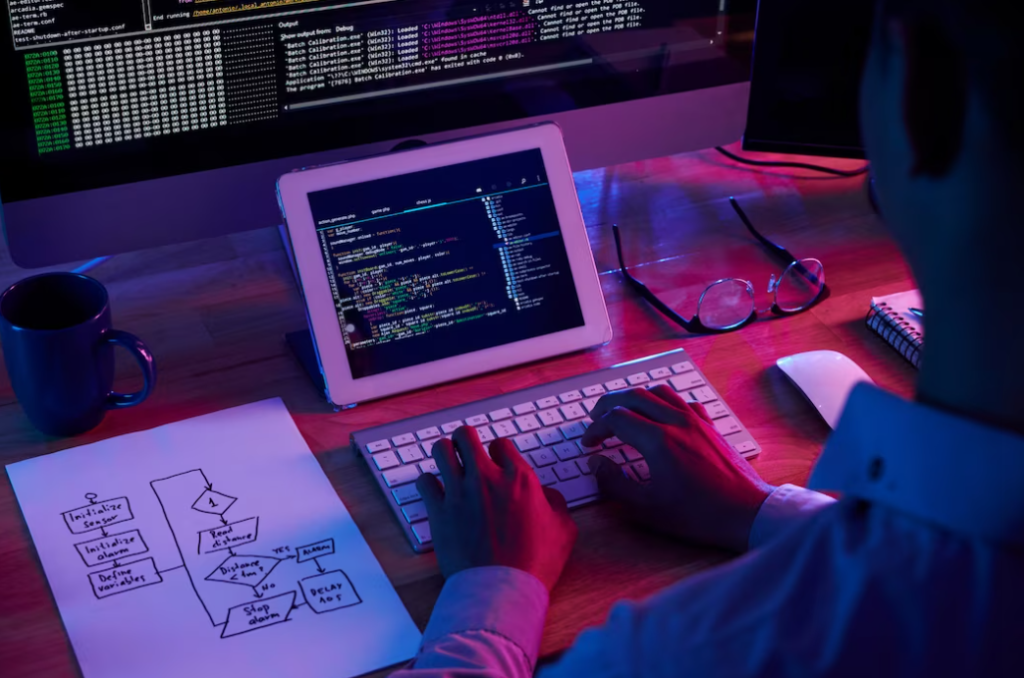
A practical guideline is to favor Node.textContent for handling straightforward text elements whenever feasible. For more intricate elements, it’s advisable to conduct an assessment of how they interact with the overall layout and user interactions. For instance, if you’re dealing with a complex element that is rendered once and remains static, HTMLElement.innerText might still be suitable. However, to mitigate potential performance concerns, consider storing the result in a variable for reuse, thereby minimizing unnecessary reflows. This nuanced approach ensures optimal performance while maintaining flexibility in your web development projects.
Conclusion
HTMLElement.innerText and Node.textContent are closely related properties designed for accessing and modifying an element’s text content. Despite their similarities, they exhibit notable distinctions that warrant attention when making your selection. It’s essential to analyze your specific use case and also take into account the potential performance impact of your choice.
Understanding Truthy and Falsy Values in JavaScript
JavaScript offers two properties for accessing the textual content within an element: Node.textContent and HTMLElement.innerText. These properties may seem interchangeable, and indeed, many developers use them interchangeably without realizing the significant distinctions between the two. Similarities Examining the similarities between these two properties before delving into their differences can provide valuable insights. This approach …
A Guide to Appending Elements in JavaScript Arrays
JavaScript offers two properties for accessing the textual content within an element: Node.textContent and HTMLElement.innerText. These properties may seem interchangeable, and indeed, many developers use them interchangeably without realizing the significant distinctions between the two. Similarities Examining the similarities between these two properties before delving into their differences can provide valuable insights. This approach …